帮我写代码实现二叉树的创建、二叉树的递归和非递归遍历
时间: 2023-10-24 11:08:47 浏览: 100
好的,以下是二叉树的创建、递归和非递归遍历的代码实现:
```python
# 定义二叉树节点类
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
# 递归创建二叉树
def create_binary_tree(nums, index):
if index >= len(nums) or nums[index] is None:
return None
# 创建当前节点
root = TreeNode(nums[index])
# 创建左子树
root.left = create_binary_tree(nums, 2 * index + 1)
# 创建右子树
root.right = create_binary_tree(nums, 2 * index + 2)
return root
# 递归遍历二叉树
def recursive_traversal(root):
if root is None:
return
# 先序遍历
print(root.val, end=' ')
recursive_traversal(root.left)
# 中序遍历
# print(root.val, end=' ')
recursive_traversal(root.right)
# 后序遍历
# print(root.val, end=' ')
# 非递归遍历二叉树
def iterative_traversal(root):
if root is None:
return
stack = []
node = root
while stack or node:
while node:
stack.append(node)
node = node.left
node = stack.pop()
# 先序遍历
print(node.val, end=' ')
node = node.right
# 中序遍历
# print(node.val, end=' ')
# node = node.right
# 后序遍历
# if node.right is not None:
# stack.append(node.right)
# stack.append(node)
# node = node.left
print()
```
其中,`create_binary_tree`函数用于递归创建二叉树,参数`nums`是按照完全二叉树的顺序存储的节点值,用`None`表示空节点;参数`index`表示当前节点在`nums`数组中的索引。`recursive_traversal`函数用于递归遍历二叉树,`iterative_traversal`函数用于非递归遍历二叉树,分别实现了先序遍历、中序遍历和后序遍历。可以根据需要选择不同的遍历方式。
阅读全文
相关推荐
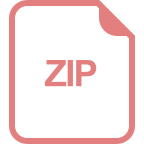
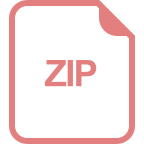
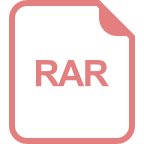
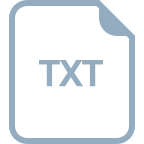
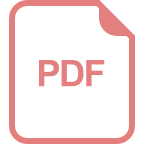
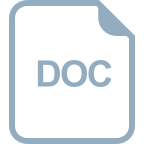
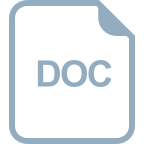
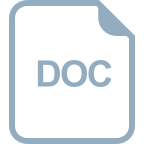


