python 读取mysql数据库的每一张表格,给每张表格增加两列,列名称为a和b,数据类型为小数.,如果a和b列存在则跳过,并且使用多线程
时间: 2023-12-19 10:06:49 浏览: 128
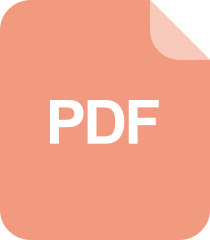
Python如何读取MySQL数据库表数据
可以使用 Python 中的 `mysql-connector-python` 库来操作 MySQL 数据库,使用 `con.cursor().execute()` 方法执行 SQL 语句。多线程可以使用 `threading` 模块来实现。
下面是一个大致的实现思路:
1. 连接到 MySQL 数据库,获取所有表格名称。
2. 对于每个表格,查询其字段信息,判断是否已经存在 a 和 b 两列。如果不存在,则使用 ALTER TABLE 语句增加这两列。
3. 使用多线程加快处理速度。
下面是一个示例代码:
```python
import mysql.connector
import threading
# 定义数据库连接信息
config = {
'user': 'root',
'password': '123456',
'host': 'localhost',
'database': 'test'
}
# 获取所有表格名称
def get_table_names(cursor):
cursor.execute("SHOW TABLES")
return [table[0] for table in cursor]
# 获取表格字段信息
def get_table_columns(cursor, table_name):
cursor.execute(f"DESC {table_name}")
return [column[0] for column in cursor]
# 判断是否存在 a 和 b 两列
def has_ab_columns(columns):
return 'a' in columns and 'b' in columns
# 增加 a 和 b 两列
def add_ab_columns(cursor, table_name):
cursor.execute(f"ALTER TABLE {table_name} ADD COLUMN a DECIMAL(10, 2), ADD COLUMN b DECIMAL(10, 2)")
# 处理单个表格
def process_table(table_name):
conn = mysql.connector.connect(**config)
cursor = conn.cursor()
columns = get_table_columns(cursor, table_name)
if not has_ab_columns(columns):
add_ab_columns(cursor, table_name)
cursor.close()
conn.close()
# 多线程处理所有表格
def process_all_tables():
conn = mysql.connector.connect(**config)
cursor = conn.cursor()
table_names = get_table_names(cursor)
cursor.close()
conn.close()
threads = []
for table_name in table_names:
thread = threading.Thread(target=process_table, args=(table_name,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
if __name__ == '__main__':
process_all_tables()
```
在这个示例代码中,我们定义了 `config` 变量来保存数据库连接信息。在 `get_table_names()` 函数中,我们使用 `SHOW TABLES` 语句获取所有表格名称。在 `get_table_columns()` 函数中,我们使用 `DESC` 语句查询表格字段信息。在 `has_ab_columns()` 函数中,我们判断是否已经存在 a 和 b 两列。在 `add_ab_columns()` 函数中,我们使用 `ALTER TABLE` 语句增加这两列。在 `process_table()` 函数中,我们根据表格名称处理单个表格。在 `process_all_tables()` 函数中,我们使用多线程处理所有表格。
注意,在多线程中,每个线程都需要连接到数据库,因此可以考虑使用连接池来提高性能,并且需要注意线程安全的问题。
阅读全文
相关推荐




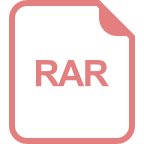
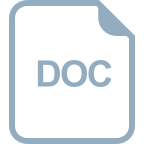
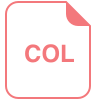
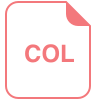
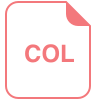
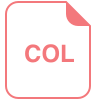
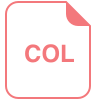
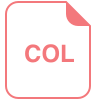
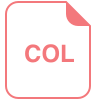
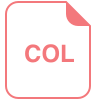
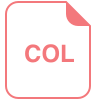
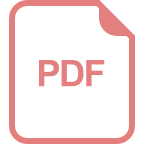