在处理有向图时,如何实现拓扑排序并找出关键路径?请结合示例代码进行说明。
时间: 2024-11-02 09:27:11 浏览: 36
在图论中,拓扑排序和关键路径分析是理解和处理项目依赖关系的重要工具。对于有向无环图(DAG),拓扑排序可以帮助我们确定任务的执行顺序,而关键路径则能帮助我们识别项目完成的最短时间路径。
参考资源链接:[图的抽象数据类型与数据结构详解](https://wenku.csdn.net/doc/7vzctfbo3x?spm=1055.2569.3001.10343)
为了实现拓扑排序,我们可以采用深度优先搜索(DFS)算法。通过递归遍历每个顶点的邻接点,并将访问过的顶点存入栈中,最终实现顶点的排序。而关键路径的求解通常基于拓扑排序的前序顶点和后序顶点进行。
以下是一个用Python实现的拓扑排序与关键路径分析的示例代码,建议结合《图的抽象数据类型与数据结构详解》进行深入学习:
```python
# 假设图使用邻接表表示
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
# 拓扑排序函数
def topological_sort(graph):
stack = []
visited = set()
def dfs(v):
visited.add(v)
for neighbour in graph[v]:
if neighbour not in visited:
dfs(neighbour)
stack.insert(0, v)
for node in graph:
if node not in visited:
dfs(node)
return stack
# 关键路径函数
def critical_path(graph):
# 拓扑排序结果
topo_order = topological_sort(graph)
# 计算最早开始时间和最晚开始时间
earliest = {v: 0 for v in graph}
latest = {v: float('inf') for v in graph}
# 从拓扑排序结果的最后一个顶点开始计算最晚开始时间
last_node = topo_order[-1]
latest[last_node] = earliest[last_node] # 最晚开始时间等于最早开始时间
# 递归计算
def calculate(node):
for neighbour in graph[node]:
calculate(neighbour)
earliest[neighbour] = max(earliest[neighbour], earliest[node] + 1)
latest[neighbour] = min(latest[neighbour], latest[node] - 1)
calculate(last_node)
# 找出关键路径
critical_path = []
for node in topo_order:
if earliest[node] == latest[node]:
critical_path.append(node)
return critical_path
# 执行拓扑排序
sorted_nodes = topological_sort(graph)
print(
参考资源链接:[图的抽象数据类型与数据结构详解](https://wenku.csdn.net/doc/7vzctfbo3x?spm=1055.2569.3001.10343)
阅读全文
相关推荐
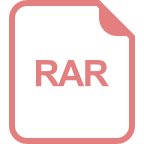
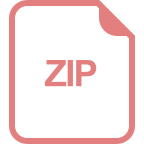
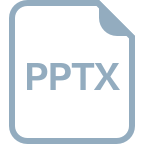

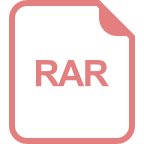
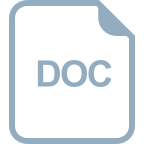
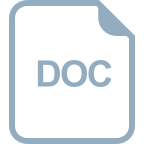
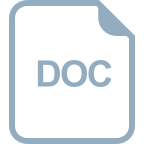
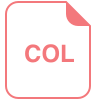
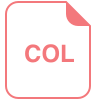
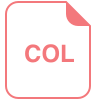
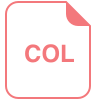
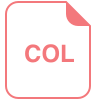
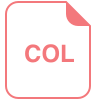
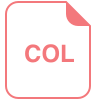
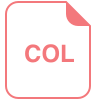
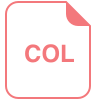
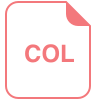
