multi head attention原理
时间: 2023-09-18 07:05:59 浏览: 129
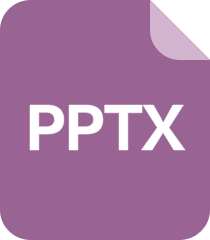
Attention_self attention_multi head attention
Multi-head attention是一种在Transformer模型中广泛使用的自注意力机制。它允许模型同时关注不同的位置和表示层次,以捕捉更丰富的语义信息。
Multi-head attention的原理如下:
1. 对输入进行线性映射
通过将输入向量乘以权重矩阵,得到三个不同的向量:query、key和value。
2. 分成多个头
将这三个向量分别切分成多个头,每个头的维度相同。
3. 计算注意力分数
对于每个头,通过计算query和key之间的点积,得到该头的注意力分数。注意力分数体现了query和key之间的相似度。
4. 归一化注意力分数
为了保证注意力分数的值在[0,1]之间,需要将注意力分数进行softmax计算,得到每个头的注意力权重。
5. 加权求和
将每个头的value向量乘以对应的注意力权重,然后将所有加权后的向量相加,得到最终的输出向量。
通过将注意力机制分成多个头,模型可以对不同的信息进行并行处理,提高了模型的并行性和表达能力。同时,由于每个头只关注部分信息,模型可以更加准确地捕捉输入的语义信息。
阅读全文
相关推荐















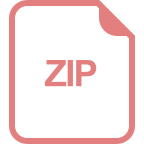
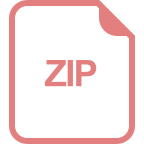