代码的运行页面#include <iostream>#include <fstream>using namespace std;int main(int argc, char *argv[]){ // 检查命令行参数 if (argc != 4) { cout << "Usage: combine file1 file2 file3" << endl; return 1; } // 打开文件1 ifstream file1(argv[1], ios::binary); if (!file1) { cout << "Cannot open " << argv[1] << endl; return 1; } // 打开文件2 ifstream file2(argv[2], ios::binary); if (!file2) { cout << "Cannot open " << argv[2] << endl; return 1; } // 创建文件3 ofstream file3(argv[3], ios::binary); if (!file3) { cout << "Cannot create " << argv[3] << endl; return 1; } // 将文件1的内容写入文件3 char c; while (file1.get(c)) { file3.put(c); } // 将文件2的内容写入文件3 while (file2.get(c)) { file3.put(c); } // 关闭文件 file1.close(); file2.close(); file3.close(); cout << "Done." << endl; return 0;}
时间: 2024-01-25 16:02:21 浏览: 18
这是一个 C++ 程序,用于将两个二进制文件合并成一个文件。它需要接受三个命令行参数,分别是要合并的第一个文件名、第二个文件名以及合并后的文件名。程序首先检查命令行参数的个数,如果不是 4 个则输出使用说明并退出程序。然后尝试打开第一个文件和第二个文件,如果打开失败则输出错误信息并退出程序。接着创建一个新的文件用于存储合并后的内容。程序使用两个 while 循环,将第一个文件和第二个文件的内容依次写入到新文件中。最后关闭所有文件,输出完成信息并退出程序。
相关问题
#include "stdafx.h" #include <windows.h> #include <process.h> #include <iostream> #include <fstream> using namespace std; HANDLE evRead, evFinish; void ReadThread(LPVOID param) { WaitForSingleObject (evRead ,INFINITE); cout<<"Reading"<<endl; SetEvent (evFinish); } void WriteThread(LPVOID param) { cout<<"Writing"<<endl; SetEvent (evRead); } int main(int argc , char * argv[]) { evRead = CreateEvent (NULL ,FALSE ,FALSE ,NULL) ; evFinish = CreateEvent (NULL ,FALSE ,FALSE ,NULL) ; _beginthread(ReadThread , 0 , NULL) ; _beginthread(WriteThread , 0 , NULL) ; WaitForSingleObject (evFinish,INFINITE) ; cout<<"The Program is End"<<endl; return 0 ; }用信号量代替事件实现线程间同步
以下是使用信号量代替事件实现线程间同步的代码:
#include <windows.h>
#include <process.h>
#include <iostream>
#include <fstream>
using namespace std;
HANDLE hSemaphore;
int counter = 0;
void ReadThread(LPVOID param) {
WaitForSingleObject(hSemaphore, INFINITE);
cout << "Reading" << endl;
ReleaseSemaphore(hSemaphore, 1, NULL);
}
void WriteThread(LPVOID param) {
WaitForSingleObject(hSemaphore, INFINITE);
cout << "Writing" << endl;
ReleaseSemaphore(hSemaphore, 1, NULL);
}
int main(int argc, char* argv[]) {
hSemaphore = CreateSemaphore(NULL, 1, 1, NULL);
_beginthread(ReadThread, 0, NULL);
_beginthread(WriteThread, 0, NULL);
WaitForSingleObject(hSemaphore, INFINITE);
cout << "The Program is End" << endl;
ReleaseSemaphore(hSemaphore, 1, NULL);
CloseHandle(hSemaphore);
return 0;
}
在这个示例中,我们使用了一个计数器和一个信号量来进行线程间同步。计数器的初始值为1,信号量的初始值也为1。在每个线程中,我们先等待信号量(相当于等待事件),然后进行操作,最后释放信号量(相当于设置事件)。在主线程中,我们先等待信号量,然后输出程序结束的信息,最后释放信号量。这样就保证了两个线程的顺序执行。
#include <iostream> #include <fstream> #include <stdlib.h> #include <cassert> #include <string> using namespace std; void printTxt(string file); int main(int argc, char* argv[]) { //路径名不能有空格 argv 字符串数组 二维数组 string filename = argv[1]; printTxt(filename); return 0; } void printTxt(string file) { ifstream infile; infile.open(file.data()); //将文件流对象与文件连接起来 assert(infile.is_open()); //若失败,则输出错误消息,并终止程序运行 string c; int i = 0; while (true) { i++; getline(infile, c); if (infile.eof()) break; cout << i << ":" << c << endl; } infile.close(); //关闭文件输入流 }改正以上代码
在上述代码中,需要加上头文件 `#include <string>`。同时,需要在函数 `printTxt` 中加上判断输入文件是否存在的语句,例如:
```c++
void printTxt(string file) {
ifstream infile(file);
if (!infile) {
cerr << "Error: Failed to open file: " << file << endl;
exit(1);
}
...
}
```
同时,在主函数中需要判断命令行参数是否足够,例如:
```c++
int main(int argc, char* argv[]) {
if (argc < 2) {
cerr << "Error: Missing command line argument for input file." << endl;
exit(1);
}
...
}
```
这样做可以确保程序在处理文件时不会出现错误。
相关推荐
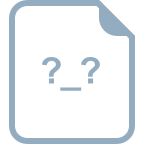













