int SetUpTCPtoSendInformation(char option[], char *command) { static int isFirstCall = 1; pthread_mutex_lock(&mutex); // 加锁 if (isFirstCall && strstr(option, "set")) { TCPCommand = malloc(strlen(command) + 1); if (TCPCommand == NULL) { CON_LOG("Failed to set and obtain TCP command variable memory allocation"); goto fail; } strcpy(TCPCommand, command); CON_LOG("set:%s",TCPCommand); isFirstCall = 0; goto succeed; } else if (!isFirstCall && strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) { free(command); command = malloc(strlen(TCPCommand) + 1); strcpy(command, TCPCommand); CON_LOG("get:%s",command); memset(TCPCommand, '\0', strlen(TCPCommand)); free(TCPCommand); isFirstCall = 1; goto succeed; } memset(command, 0, sizeof(command)); strcpy(command, ""); fail: pthread_mutex_unlock(&mutex); // 解锁 return 0; succeed: pthread_mutex_unlock(&mutex); // 解锁 return 1; } SetUpTCPtoSendInformation("set","sssssss"); char *resultbuf =NULL; SetUpTCPtoSendInformation("get",resultbuf); char *a; free(a); a=malloc(strlen(resultbuf) + 1); strcpy(a,resultbuf); printf("%d %s",strlen(resultbuf),a);帮检查一下这样调用有误么,我要a和b都要拿到set的值,如果有误请帮我修改
时间: 2024-04-10 12:33:30 浏览: 93
你的代码存在一些问题。首先,在 `SetUpTCPtoSendInformation` 函数中,`command` 参数应该是一个指向字符数组的指针,而不是字符数组本身。因此,你需要修改函数的定义为 `int SetUpTCPtoSendInformation(char option[], char **command)`,并使用 `*command` 来访问和修改传入的指针。
其次,在调用函数 `SetUpTCPtoSendInformation("get", resultbuf)` 时,你将 `resultbuf` 传递给函数,但这个指针并没有被分配内存。你需要在调用之前将其分配内存,例如 `resultbuf = malloc(sizeof(char) * MAX_LENGTH)`,其中 `MAX_LENGTH` 是你预先定义的足够大的值。
最后,在第二个调用中,你需要将 `resultbuf` 的内容复制到指针 `a` 指向的内存中。因此,你需要修改代码为 `strcpy(a, resultbuf)`。
以下是修改后的代码示例:
```c
int SetUpTCPtoSendInformation(char option[], char **command) {
static int isFirstCall = 1;
pthread_mutex_lock(&mutex); // 加锁
if (isFirstCall && strstr(option, "set")) {
TCPCommand = malloc(strlen(*command) + 1);
if (TCPCommand == NULL) {
CON_LOG("Failed to set and obtain TCP command variable memory allocation");
goto fail;
}
strcpy(TCPCommand, *command);
CON_LOG("set:%s", TCPCommand);
isFirstCall = 0;
goto succeed;
} else if (!isFirstCall && strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) {
free(*command);
*command = malloc(strlen(TCPCommand) + 1);
strcpy(*command, TCPCommand);
CON_LOG("get:%s", *command);
memset(TCPCommand, '\0', strlen(TCPCommand));
free(TCPCommand);
isFirstCall = 1;
goto succeed;
}
memset(*command, 0, sizeof(*command));
strcpy(*command, "");
fail:
pthread_mutex_unlock(&mutex); // 解锁
return 0;
succeed:
pthread_mutex_unlock(&mutex); // 解锁
return 1;
}
char *resultbuf = NULL;
resultbuf = malloc(sizeof(char) * MAX_LENGTH);
SetUpTCPtoSendInformation("get", &resultbuf);
char *a = NULL;
a = malloc(strlen(resultbuf) + 1);
strcpy(a, resultbuf);
printf("%d %s", strlen(resultbuf), a);
```
请确保在使用 `resultbuf` 和 `a` 变量之后,使用 `free` 函数释放它们所分配的内存。同时,根据你的需求,你可能需要修改 `MAX_LENGTH` 的值以确保分配了足够的内存。
阅读全文
相关推荐
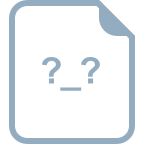
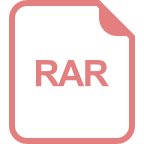
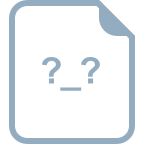







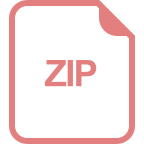
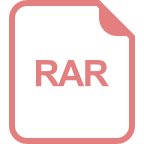
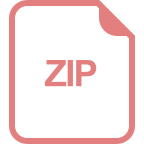
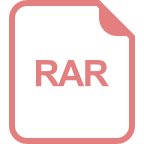
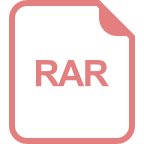
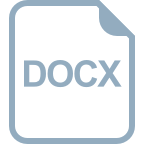