用switch嵌套循环写一个点餐系统
时间: 2024-09-21 11:08:05 浏览: 47
在编写一个点餐系统,特别是使用switch嵌套循环的方式时,我们可以设想一个简单的场景,比如用户可以选择菜单项(例如主菜、配菜、饮料),然后针对每个选择再提供几个选项。这里是一个基础示例:
```java
import java.util.Scanner;
public class MenuSystem {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int choice;
// 主菜单
System.out.println("欢迎来到点餐系统,请选择:");
System.out.println("1. 主菜");
System.out.println("2. 配菜");
System.out.println("3. 饮料");
System.out.print("请输入您的选择: ");
choice = input.nextInt();
switch (choice) {
case 1: // 主菜
switch (getSubChoice("请选择主菜:", new String[]{"牛肉", "鸡肉", "鱼肉"})) {
case 0:
break; // 没有选择或已结束
default:
System.out.println("您选择了: " + getSubChoiceOptions(choice - 1)[getSubChoice()]);
break;
}
break;
case 2: // 配菜
switch (getSubChoice("请选择配菜:", new String[]{"炒饭", "面条", "沙拉"})) {
case 0:
break;
default:
System.out.println("您选择了: " + getSubChoiceOptions(choice - 1)[getSubChoice()]);
break;
}
break;
case 3: // 饮料
switch (getSubChoice("请选择饮料:", new String[]{"可乐", "果汁", "茶"})) {
case 0:
break;
default:
System.out.println("您选择了: " + getSubChoiceOptions(choice - 1)[getSubChoice()]);
break;
}
break;
default:
System.out.println("无效的选择,请从1到3");
}
input.close();
}
private static int getSubChoice(String prompt, String[] options) {
while (true) {
System.out.print(prompt);
for (int i = 0; i < options.length; i++) {
System.out.println((i+1) + ". " + options[i]);
}
System.out.print("请输入数字选择: ");
int subChoice = input.nextInt() - 1;
if (subChoice >= 0 && subChoice < options.length) {
return subChoice;
} else {
System.out.println("无效的选择,请重试.");
}
}
}
private static String[] getSubChoiceOptions(int mainChoice) {
// 这里可以添加根据主菜不同,返回对应的配菜或饮料列表
// 示例:
// 如果mainChoice是1(主菜),那么就返回一些配菜选项,如 {"炒饭", "面条"}
// 类似地,如果mainChoice是2或3,返回对应饮料选项
return new String[0]; // 真实项目中需要填充实际数据
}
}
```
这个程序会提示用户选择主菜、配菜或饮料,并根据用户输入调用不同的子菜单。每个`getSubChoice`方法内还有进一步的循环,用于细化各个子分类的选择。
阅读全文
相关推荐
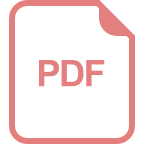
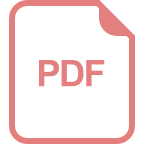
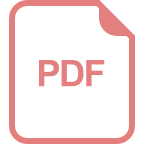















