定义An array often pointers to integers
时间: 2024-02-25 13:55:31 浏览: 96
可以使用如下语法定义一个包含10个整型变量指针的数组:
```
int* arr[10];
```
其中,arr是一个包含10个整型变量指针的数组,每一个元素都是一个指向整型变量的指针,可以通过它们访问指向的整型变量,并修改其值。需要注意的是,这里并没有初始化指针数组中的每一个元素,所以在使用之前需要先初始化。
相关问题
Given an array of positive integers nums and a positive integer target, return the minimal length of a subarray whose sum is greater than or equal to target. If there is no such subarray, return 0 instead.
This problem can be solved using the sliding window technique. We maintain a window of elements whose sum is greater than or equal to the target. We start with two pointers, left and right, both pointing to the first element of the array. We then move the right pointer to the right until the sum of elements in the window is greater than or equal to the target. Once we have found such a window, we update the answer with the length of the current window. We then move the left pointer to the right until the sum of elements in the window is less than the target. We repeat this process until the right pointer reaches the end of the array. We return the answer.
Here is the Python code:
```
def minSubArrayLen(nums, target):
n = len(nums)
left = right = 0
window_sum = 0
ans = float('inf')
while right < n:
window_sum += nums[right]
while window_sum >= target:
ans = min(ans, right - left + 1)
window_sum -= nums[left]
left += 1
right += 1
return ans if ans != float('inf') else 0
```
The time complexity of this algorithm is O(n) and the space complexity is O(1).
Write c++ program. Use Pointers to write a function to bubble sort an integer array. Input format. Specifies the length of the sequence and enters the sequence. Output format. Output in order from smallest to largest
Sure, here's the C++ program to bubble sort an integer array using pointers:
```c++
#include <iostream>
using namespace std;
void bubbleSort(int *arr, int n) {
for(int i=0; i<n-1; i++) {
for(int j=0; j<n-i-1; j++) {
if(*(arr+j) > *(arr+j+1)) {
int temp = *(arr+j);
*(arr+j) = *(arr+j+1);
*(arr+j+1) = temp;
}
}
}
}
int main() {
int n;
cout << "Enter the length of the sequence: ";
cin >> n;
int arr[n];
cout << "Enter the sequence: ";
for(int i=0; i<n; i++) {
cin >> *(arr+i);
}
bubbleSort(arr, n);
cout << "The sorted sequence is: ";
for(int i=0; i<n; i++) {
cout << *(arr+i) << " ";
}
return 0;
}
```
In this program, we first take input the length of the integer array and then the sequence itself. We pass this array and its length to the bubbleSort() function that implements the bubble sorting algorithm using pointers. Finally, we output the sorted sequence.
阅读全文
相关推荐


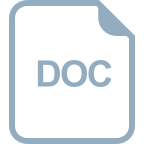













