Write a function RemoveDuplicates (array, n) that removes all duplicatevalues from a sorted array of integers, leaving only a single copy of each value.RemoveDuplicates should return the effective size of the new array.
时间: 2024-03-06 19:51:03 浏览: 15
Sure, here's a possible implementation of the RemoveDuplicates function in C++:
```
int RemoveDuplicates(int array[], int n) {
if (n == 0) {
return 0;
}
int j = 0;
for (int i = 1; i < n; i++) {
if (array[i] != array[j]) {
j++;
array[j] = array[i];
}
}
return j + 1;
}
```
The function takes as input an array of integers `array` and its size `n`. It assumes that the array is sorted in non-decreasing order, so that duplicates are adjacent. The function then uses two pointers `i` and `j` to scan the array, keeping only the first occurrence of each value. The variable `j` keeps track of the last non-duplicate value found, and is used to overwrite the duplicates with new values. Finally, the function returns the size of the new array, which is `j+1`, since `j` is the index of the last non-duplicate value.
Here's an example of how to use the function:
```
int main() {
int array[] = {1, 2, 2, 3, 3, 3, 4, 5, 5, 5, 5, 6};
int n = sizeof(array) / sizeof(array[0]);
int new_size = RemoveDuplicates(array, n);
for (int i = 0; i < new_size; i++) {
std::cout << array[i] << " ";
}
std::cout << std::endl;
return 0;
}
```
This should output:
```
1 2 3 4 5 6
```
相关推荐
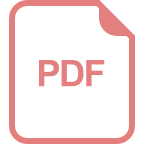
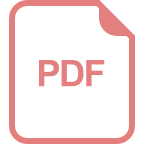
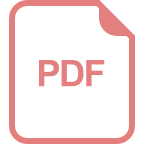















