(Remove duplicates) Write a method that removes the duplicate elements from an array list of integers using the following header: public static void removeDuplicate(ArrayList<Integer> list) Write a test program that prompts the user to enter 10 integers to a list and displays the distinct integers in their input order and separated by exactly one space. Here is a sample run: Enter 10 integers: 34 5 3 5 6 4 33 2 2 4 The distinct integers are 34 5 3 6 4 33 2
时间: 2023-11-22 17:53:09 浏览: 82
Here's the implementation of the `removeDuplicate` method:
```java
public static void removeDuplicate(ArrayList<Integer> list) {
ArrayList<Integer> distinctList = new ArrayList<Integer>();
for (Integer num : list) {
if (!distinctList.contains(num)) {
distinctList.add(num);
}
}
list.clear();
list.addAll(distinctList);
}
```
And here's the test program:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
ArrayList<Integer> list = new ArrayList<Integer>();
System.out.print("Enter 10 integers: ");
for (int i = 0; i < 10; i++) {
int num = input.nextInt();
list.add(num);
}
removeDuplicate(list);
System.out.print("The distinct integers are ");
for (Integer num : list) {
System.out.print(num + " ");
}
}
public static void removeDuplicate(ArrayList<Integer> list) {
ArrayList<Integer> distinctList = new ArrayList<Integer>();
for (Integer num : list) {
if (!distinctList.contains(num)) {
distinctList.add(num);
}
}
list.clear();
list.addAll(distinctList);
}
}
```
Sample output:
```
Enter 10 integers: 34 5 3 5 6 4 33 2 2 4
The distinct integers are 34 5 3 6 4 33 2
```
阅读全文
相关推荐
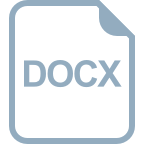
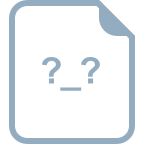
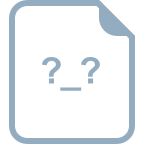
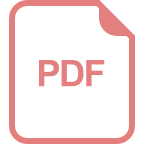
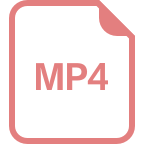












