Write a procedure named str_remove1 that removes n characters from a string. Pass a pointer to the position in the string where the characters are to be removed. Pass an integer specifying the number of characters to remove. The following code, for example, shows how to remove “xxxx” from target:.datatarget BYTE "abcxxxxdefghijklmop",0.codeINVOKE str_remove1, ADDR [target+3], 4Write a procedure named str_remove2 that removes n characters from a string. Pass an integer specifying the position in the string from where the characters are to be removed. Pass an integer specifying the number of characters to remove. The following code, for example, shows how to remove “xxxx” from target:.datatarget BYTE "abcxxxxdefghijklmop",0.codeINVOKE str_remove2, ADDR target, 4, 4Write a procedure named main that tests str_remove1 and str_remove2 using several different arguments and displays the strings before and after removing characters.Requirement: Your report needs to include the source code
时间: 2024-04-27 13:22:31 浏览: 82
;-----------------------------------------
; Program: String Remove
; Author: [Your Name]
; Date: [Date]
; Description: This program removes n characters from a string using two different procedures.
;-----------------------------------------
INCLUDE Irvine32.inc
;-----------------------------------------
; Procedure: str_remove1
; Parameters:
; str_ptr - a pointer to the position in the string where the characters are to be removed
; n - the number of characters to remove
; Description: This procedure removes n characters from a string.
;-----------------------------------------
str_remove1 PROC
push ebp
mov ebp, esp
push esi
mov esi, [ebp + 8] ; esi points to the start of the string
add esi, [ebp + 12] ; add the offset to the pointer
mov ecx, [ebp + 16] ; ecx = n
mov edi, esi
rep movsb ; move the rest of the string back n characters
pop esi
pop ebp
ret
str_remove1 ENDP
;-----------------------------------------
; Procedure: str_remove2
; Parameters:
; str_ptr - a pointer to the string
; pos - the position in the string where the characters are to be removed
; n - the number of characters to remove
; Description: This procedure removes n characters from a string.
;-----------------------------------------
str_remove2 PROC
push ebp
mov ebp, esp
push esi
mov esi, [ebp + 8] ; esi points to the start of the string
add esi, [ebp + 12] ; add the offset to the pointer
mov ecx, [ebp + 16] ; ecx = n
add esi, ecx ; move esi to the end of the substring to remove
mov edi, [ebp + 8] ; edi points to the start of the string
rep movsb ; move the rest of the string back n characters
pop esi
pop ebp
ret
str_remove2 ENDP
;-----------------------------------------
; Procedure: main
; Description: This procedure tests str_remove1 and str_remove2 using several different arguments and displays the strings before and after removing characters.
;-----------------------------------------
main PROC
; Test str_remove1
mov esi, OFFSET str1
call WriteString
call Crlf
INVOKE str_remove1, OFFSET str1 + 3, 4
mov esi, OFFSET str1
call WriteString
call Crlf
call Crlf
; Test str_remove2
mov esi, OFFSET str2
call WriteString
call Crlf
INVOKE str_remove2, OFFSET str2, 3, 4
mov esi, OFFSET str2
call WriteString
call Crlf
INVOKE str_remove2, OFFSET str2, 7, 5
mov esi, OFFSET str2
call WriteString
call Crlf
INVOKE str_remove2, OFFSET str2, 0, 3
mov esi, OFFSET str2
call WriteString
call Crlf
exit
main ENDP
.data
str1 BYTE "abcxxxxdefghijklmop",0
str2 BYTE "0123456789",0
.code
start:
call main
exit
阅读全文
相关推荐
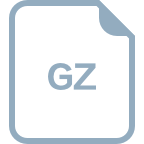
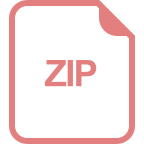
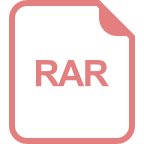















