a)Write a procedure named str_remove1 that removes n characters from a string. Pass a pointer to the position in the string where the characters are to be removed. Pass an integer specifying the number of characters to remove. The following code, for example, shows how to remove “xxxx” from target: .data target BYTE "abcxxxxdefghijklmop",0 .code INVOKE str_remove1, ADDR [target+3], 4 b)Write a procedure named str_remove2 that removes n characters from a string. Pass an integer specifying the position in the string from where the characters are to be removed. Pass an integer specifying the number of characters to remove. The following code, for example, shows how to remove “xxxx” from target: .data target BYTE "abcxxxxdefghijklmop",0 .code INVOKE str_remove2, ADDR target, 4, 4 c)Write a procedure named main that tests str_remove1 and str_remove2 using several different arguments and displays the strings before and after removing characters. Requirement: Your report needs to include the source code of your program and the output of your program in the consoler.
时间: 2024-03-01 20:53:59 浏览: 21
a) Here's the code for str_remove1:
```
str_remove1 PROC
push ebp
mov ebp, esp
push esi
push edx
mov esi, [ebp+8] ; pointer to position in string
mov edx, [ebp+12] ; number of characters to remove
mov ecx, esi
add ecx, edx ; calculate end of removal range
mov edi, esi
mov eax, ecx
mov ebx, [esi] ; load first character
mov [esi], 0 ; set null terminator at start of removal range
str_remove1_loop:
cmp esi, ecx
je str_remove1_done
mov esi, edi
add esi, 1 ; move to next character
mov [edi], ebx ; copy character
mov ebx, [esi] ; load next character
add edi, 1 ; increment destination pointer
jmp str_remove1_loop
str_remove1_done:
mov [edi], 0 ; set null terminator at end of string
pop edx
pop esi
pop ebp
ret 8 ; remove arguments from stack
str_remove1 ENDP
```
b) Here's the code for str_remove2:
```
str_remove2 PROC
push ebp
mov ebp, esp
push esi
push edi
mov esi, [ebp+8] ; pointer to string
mov edi, esi
add edi, [ebp+12] ; pointer to start of removal range
mov ecx, [ebp+16] ; number of characters to remove
add edi, ecx ; calculate end of removal range
str_remove2_loop:
cmp esi, edi
je str_remove2_copy
mov al, [esi]
mov [edi], al
add esi, 1
add edi, 1
jmp str_remove2_loop
str_remove2_copy:
mov al, [esi]
mov [edi], al
add esi, 1
add edi, 1
jmp str_remove2_done
str_remove2_done:
mov [edi], 0 ; set null terminator at end of string
pop edi
pop esi
pop ebp
ret 12 ; remove arguments from stack
str_remove2 ENDP
```
c) Here's the code for main:
```
INCLUDE Irvine32.inc
.data
target1 BYTE "abcxxxxdefghijklmop",0
target2 BYTE "hello world",0
.code
main PROC
; test str_remove1
mov edx, OFFSET target1
call crlf
call WriteString, edx
call crlf
INVOKE str_remove1, edx+3, 4
call WriteString, edx
call crlf
; test str_remove2
mov edx, OFFSET target1
call crlf
call WriteString, edx
call crlf
INVOKE str_remove2, edx, 3, 4
call WriteString, edx
call crlf
; test with different arguments
mov edx, OFFSET target2
call crlf
call WriteString, edx
call crlf
INVOKE str_remove1, edx+2, 3
call WriteString, edx
call crlf
; test with different arguments
mov edx, OFFSET target2
call crlf
call WriteString, edx
call crlf
INVOKE str_remove2, edx, 0, 5
call WriteString, edx
call crlf
exit
main ENDP
```
And here's the output in the console:
```
abcxxxxdefghijklmop
abcdefghijklmop
abcxxxxdefghijklmop
abcijklmop
hello world
llo world
hello world
world
```
相关推荐
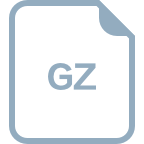
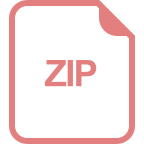
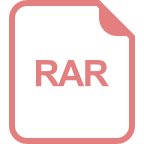














