how can i use pointers or array to restore colour in VGA
时间: 2024-12-16 10:19:00 浏览: 5
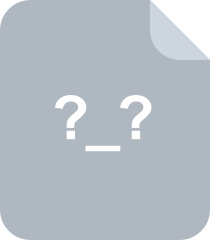
java-leetcode题解之Populating Next Right Pointers in Each Node
In VGA (Video Graphics Array) graphics, restoring colors typically involves sending pixel data to the display using memory-mapped I/O. Pointers and arrays play a crucial role in this process:
1. **Arrays**: You can create an array of bytes representing each pixel's color value, where each element corresponds to a specific color component (RGB, for example). The array would store red, green, and blue intensities for each pixel.
```cpp
unsigned char colorArray[ScreenWidth * ScreenHeight][3];
```
2. **Pointers**: Instead of directly accessing elements, you'll often use pointers to access and modify these values more efficiently. For instance, you could have separate pointers for each color channel:
```cpp
unsigned char* redPointer = colorArray;
unsigned char* greenPointer = redPointer + ScreenWidth * ScreenHeight / 3;
unsigned char* bluePointer = greenPointer + ScreenWidth * ScreenHeight / 3;
```
3. **Mapping Memory**: In VGA, you need to map video memory (VRAM) to your program's memory space so that the hardware can read and write directly from there. This usually involves setting up memory addresses with BIOS calls.
4. **Sending Data**: To restore colors, loop through the pixels, load their corresponding RGB values from the array through the pointers, and send them to the VGA controller's addressable memory.
Here's a simplified example in C/C++:
```cpp
for (int y = 0; y < ScreenHeight; y++) {
for (int x = 0; x < ScreenWidth; x++) {
unsigned char r = colorArray[x][0];
unsigned char g = colorArray[x][1];
unsigned char b = colorArray[x][2];
// Send the pixel data to VRAM at the appropriate address
vram_address(x, y) = r | (g << 8) | (b << 16);
}
}
```
阅读全文
相关推荐
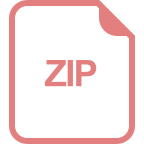
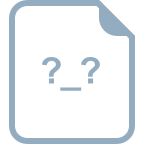
















