java五子棋代码仅输出
时间: 2023-07-09 15:34:45 浏览: 55
以下是一个简单的 Java 五子棋代码,仅输出棋盘:
```java
public class Gobang {
public static void main(String[] args) {
int[][] board = new int[15][15];
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
board[i][j] = 0;
}
}
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
}
```
注意:这个代码仅仅输出了一个棋盘,没有实现五子棋的游戏逻辑和人机对战等功能。
相关问题
java五子棋游戏代码
五子棋是一种非常古老和流行的策略棋类游戏,也是许多程序员用来练手的经典项目之一。在Java语言中,我们可以使用面向对象的思想来设计和实现五子棋游戏代码。
首先,我们需要定义一个棋盘类,用于绘制棋盘、保存棋子、判断胜负等。棋盘类包含一个二维数组用于保存棋子位置、棋子颜色、当前落子方等信息,同时也可以定义一些方法如下:
```java
public class ChessBoard {
private int[][] board;
private int currentColor;
private boolean gameOver;
// 构造方法
public ChessBoard() {
board = new int[15][15];
currentColor = 1;
gameOver = false;
}
// 落子方法
public boolean putChess(int x, int y) {
if (board[x][y] == 0) {
board[x][y] = currentColor;
if (checkWin(x, y)) {
gameOver = true;
}
currentColor = 3 - currentColor;
return true;
}
return false;
}
// 判断胜负方法
public boolean checkWin(int x, int y) {
int color = board[x][y];
int count1 = countChess(x, y, color, 1, 0);
int count2 = countChess(x, y, color, 0, 1);
int count3 = countChess(x, y, color, 1, 1);
int count4 = countChess(x, y, color, -1, 1);
if (count1 >= 5 || count2 >= 5 || count3 >= 5 || count4 >= 5) {
return true;
}
return false;
}
// 计算同色棋子数
public int countChess(int x, int y, int color, int dx, int dy) {
int count = 1;
int i = x + dx;
int j = y + dy;
while (i >= 0 && i < 15 && j >= 0 && j < 15 && board[i][j] == color) {
count++;
i += dx;
j += dy;
}
i = x - dx;
j = y - dy;
while (i >= 0 && i < 15 && j >= 0 && j < 15 && board[i][j] == color) {
count++;
i -= dx;
j -= dy;
}
return count;
}
// 绘制棋盘方法
public void paintBoard(Graphics g) {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
if (board[i][j] == 1) {
g.setColor(Color.BLACK);
g.fillOval(i*30+10, j*30+10, 20, 20);
} else if (board[i][j] == 2) {
g.setColor(Color.WHITE);
g.fillOval(i*30+10, j*30+10, 20, 20);
}
}
}
g.setColor(Color.BLACK);
for (int i = 0; i < 15; i++) {
g.drawLine(10, i*30+10, 430, i*30+10);
g.drawLine(i*30+10, 10, i*30+10, 430);
}
}
}
```
然后我们需要定义一个主程序类,用于实现用户交互、事件处理等。主程序类包含一个棋盘对象,可以监听鼠标事件来落子,同时也可以根据游戏状态输出胜负信息等。主程序类的关键代码如下:
```java
public class Gobang extends Frame implements MouseListener {
private ChessBoard chessBoard;
// 构造方法
public Gobang() {
chessBoard = new ChessBoard();
setSize(450, 450);
addMouseListener(this);
setVisible(true);
}
// 重写绘制方法
public void paint(Graphics g) {
chessBoard.paintBoard(g);
if (chessBoard.isGameOver()) {
String msg = "Game Over!";
if (chessBoard.getCurrentColor() == 1) {
msg += " Black Wins!";
} else {
msg += " White Wins!";
}
g.setColor(Color.RED);
g.setFont(new Font("Times New Roman",Font.BOLD,20));
g.drawString(msg, 150, 240);
}
}
// 鼠标事件处理
public void mouseClicked(MouseEvent e) {
if (chessBoard.isGameOver()) {
return;
}
int x = e.getX() / 30;
int y = e.getY() / 30;
if (chessBoard.putChess(x, y)) {
repaint();
}
}
public void mousePressed(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
// 主函数
public static void main(String[] args) {
new Gobang();
}
}
```
最后,我们运行主函数可以看到五子棋游戏的界面,使用鼠标落子即可。如果有一方获得了五子连珠,游戏将会结束并显示胜负信息。
java简易五子棋代码
以下是一个简单的 Java 五子棋代码示例:
```java
import java.util.Scanner;
public class Gobang {
private static int BOARD_SIZE = 15;
private String[][] board = new String[BOARD_SIZE][BOARD_SIZE];
public void initBoard() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = "+";
}
}
}
public void printBoard() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
System.out.print(board[i][j]);
}
System.out.print("\n");
}
}
public void play() {
Scanner scanner = new Scanner(System.in);
int x = 0, y = 0;
String color = "x";
boolean flag = true;
while (flag) {
System.out.println("请输入您的落子位置,格式为x,y:");
x = scanner.nextInt();
y = scanner.nextInt();
if (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE) {
System.out.println("落子位置不合法,请重新输入!");
continue;
}
if (!board[x][y].equals("+")) {
System.out.println("该位置已经有子,请重新输入!");
continue;
}
board[x][y] = color;
printBoard();
if (isWin(x, y)) {
flag = false;
System.out.println(color + "方获胜!");
}
color = color.equals("x") ? "o" : "x";
}
scanner.close();
}
public boolean isWin(int x, int y) {
String color = board[x][y];
int count = 1;
// 判断横向
for (int i = y - 1; i >= 0; i--) {
if (board[x][i].equals(color)) {
count++;
} else {
break;
}
}
for (int i = y + 1; i < BOARD_SIZE; i++) {
if (board[x][i].equals(color)) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
} else {
count = 1;
}
// 判断纵向
for (int i = x - 1; i >= 0; i--) {
if (board[i][y].equals(color)) {
count++;
} else {
break;
}
}
for (int i = x + 1; i < BOARD_SIZE; i++) {
if (board[i][y].equals(color)) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
} else {
count = 1;
}
// 判断左上右下
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0; i--, j--) {
if (board[i][j].equals(color)) {
count++;
} else {
break;
}
}
for (int i = x + 1, j = y + 1; i < BOARD_SIZE && j < BOARD_SIZE; i++, j++) {
if (board[i][j].equals(color)) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
} else {
count = 1;
}
// 判断右上左下
for (int i = x - 1, j = y + 1; i >= 0 && j < BOARD_SIZE; i--, j++) {
if (board[i][j].equals(color)) {
count++;
} else {
break;
}
}
for (int i = x + 1, j = y - 1; i < BOARD_SIZE && j >= 0; i++, j--) {
if (board[i][j].equals(color)) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
} else {
return false;
}
}
public static void main(String[] args) {
Gobang gobang = new Gobang();
gobang.initBoard();
gobang.printBoard();
gobang.play();
}
}
```
该代码实现了一个简单的五子棋游戏,包括棋盘的初始化、输出、落子、判断胜负等功能。其中,isWin()方法用于判断是否获胜,通过遍历每一个方向上的棋子个数来实现。
相关推荐
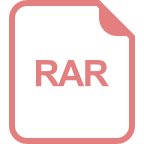
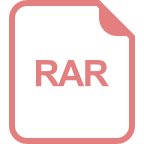
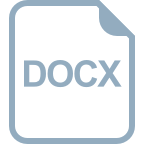












