def rts_smooth(data, window_size): data = np.array(data) m, n = data.shape smoothed_data = np.copy(data) # 创建一个与原数组相同的副本,用于存储平滑后的数据 # 遍历每一列 for j in range(n): # 使用原数据填充每一列首尾的窗口大小一半的位置 fill_value = np.concatenate((data[0, j] * np.ones(window_size // 2), data[:, j], data[-1, j] * np.ones(window_size // 2))) # 遍历每一行 for i in range(window_size // 2, m + window_size // 2): # 取窗口内的数据进行RTS平滑处理 weights = np.abs(np.arange(-window_size // 2, window_size // 2 + 1) / window_size) smoothed_data[i - window_size // 2, j] = np.sum(fill_value[i - window_size // 2:i + window_size // 2 + 1] * weights) / np.sum(weights) return smoothed_data以上代码运行出现smoothed_data[i - window_size // 2, j] = np.sum(fill_value[i - window_size // 2:i + window_size // 2 + 1] * weights) / np.sum(weights) ValueError: operands could not be broadcast together with shapes (3,) (4,)
时间: 2024-04-11 22:30:39 浏览: 136
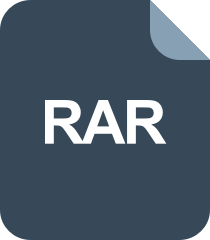
bianyuan.rar_CSharp 图像处理_smoothed_图像处理_拉普拉斯_算法 CSharp
这个错误是由于窗口大小 `window_size` 的值不正确导致的。
根据代码,窗口大小 `window_size` 应该是一个奇数,因为代码中使用了 `window_size // 2` 来确定填充值和平滑数据的位置。但是,根据错误信息,窗口大小 `window_size` 的值为偶数,导致无法正确广播数组。
请确保将窗口大小 `window_size` 设置为一个奇数,以解决这个问题。如果你想使用偶数窗口大小,可以根据具体情况修改代码适应偶数窗口的情况。例如,在计算平滑值时,可以将权重数组的长度修改为 `window_size + 1`,以匹配填充值的长度。
另外,还需要确保输入的 `data` 是一个二维数组,其中每列代表一个信号序列。如果输入的 `data` 不是二维数组,请先进行相应的处理以使其满足要求。
如果你仍然遇到问题,请提供更多关于输入数据和窗口大小的详细信息,以便更好地帮助你解决问题。
阅读全文
相关推荐
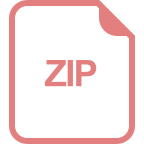
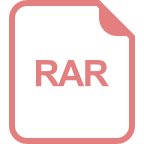
















