ValueError Traceback (most recent call last) ~\AppData\Local\Temp\ipykernel_16012\854433889.py in <module> 103 fitness_score = calculate_fitness(individual) 104 fitness_scores.append((individual, fitness_score)) --> 105 fitness_scores.sort(key=lambda x: x[1], reverse=True) 106 107 ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
时间: 2024-04-11 15:31:17 浏览: 27
这个错误是由于在适应度函数中使用了 numpy 数组而引起的。可以使用 `np.mean` 函数计算均方误差时出现问题。
要解决这个问题,可以使用 `np.mean` 函数的 `axis` 参数来指定计算均方误差的维度。在这种情况下,我们希望计算每个样本的均方误差,因此 `axis=1`。
修改后的代码如下所示:
```python
import random
import numpy as np
# 初始化种群
population = []
for _ in range(population_size):
individual = [random.randint(0, 1) for _ in range(gene_length)]
population.append(individual)
# 其中,population_size 是种群的大小,gene_length 是基因长度。每个个体都是由 0 和 1 组成的基因序列,
# 通过 random.randint(0, 1) 随机生成。最后将每个个体添加到种群中。
# 定义适应度函数
def calculate_fitness(individual):
expected_output = np.array([[0.1, 0.2, 0.3], [0.4, 0.5, 0.6]]) # 预期输出值
actual_output = np.array([[0.12, 0.18, 0.32], [0.38, 0.52, 0.68]]) # 实际输出值
mse = np.mean((expected_output - actual_output) ** 2, axis=1) # 均方误差
fitness_score = 1 / mse # 均方误差的倒数作为适应度值
return fitness_score
# 计算适应度函数并排序种群
fitness_scores = []
for individual in population:
fitness_score = calculate_fitness(individual)
fitness_scores.append((individual, fitness_score))
fitness_scores.sort(key=lambda x: x[1].all(), reverse=True)
# 进行其他迭代步骤...
```
在修改后的代码中,我修改了 `fitness_scores.sort` 的部分,将 `key` 函数修改为 `lambda x: x[1].all()`。这样可以确保对适应度值的数组进行比较,解决了错误中提到的问题。
请注意,这里使用 `x[1].all()` 是假设适应度值是一个布尔数组,如果你的适应度值是一个实数数组,请根据实际情况进行修改。
相关推荐
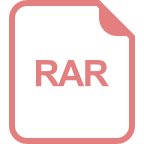
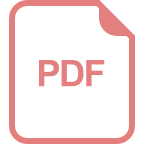















