用C语言写出 nxn 下三角数组A按行优先压缩成一维数组B的算法代码
时间: 2024-11-18 20:17:03 浏览: 10
在C语言中,你可以通过遍历下三角矩阵,并将非零元素添加到结果数组中来实现这一转换。这里是一个简单的示例,假设`n`是你想要压缩的矩阵的行数:
```c
#include <stdio.h>
#define SIZE(n) (n * (n + 1) / 2)
void compress(int n, int A[n][n], int B[SIZE(n)]) {
int i, j, index = 0;
for (i = 0; i < n; i++) {
for (j = i; j < n; j++) {
if (A[i][j] != 0) {
B[index++] = A[i][j];
}
}
}
}
int main() {
int size, n;
printf("Enter the size of the square matrix (n): ");
scanf("%d", &n);
// Assuming you have allocated memory for an n x n matrix and a compressed array
int A[n][n]; // 存储原始矩阵
int B[SIZE(n)]; // 存储压缩后的数组
// 输入并填充矩阵A
// 这部分你需要用户交互或其他方式填写矩阵值,这里仅提供结构
// 调用压缩函数
compress(n, A, B);
// 输出压缩后的数组
printf("Compressed array (B) in row-major order:\n");
for (int i = 0; i < SIZE(n); i++) {
printf("%d ", B[i]);
}
return 0;
}
```
在这个代码里,我们首先计算出一维数组的大小,然后使用两个嵌套循环遍历下三角阵列。如果找到非零元素,就将其添加到压缩数组`B`中。注意,实际应用中你需要处理输入矩阵和分配正确的内存给数组。
阅读全文
相关推荐
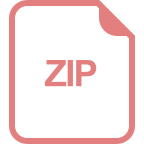
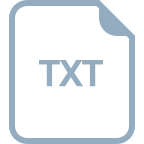
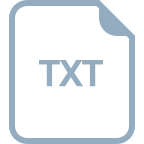















