用Java代码在WordCount基础上进行修改,能够完成以下的任务。 根据给定的输入文件,统计每个人的薪资总额。 能够在伪分布式集群和IDE环境下,实现功能。
时间: 2024-02-11 08:04:39 浏览: 15
好的,这是一个关于Hadoop的MapReduce编程的问题。首先需要在本地或者虚拟机上安装Hadoop环境,并且在IDE中配置好Hadoop相关的jar包和环境变量。
然后,需要编写Mapper和Reducer类。Mapper类将输入文件中的每一行转换为一个键值对,其中键是人名,值是薪资。Reducer类将相同键的值进行累加,得到每个人的薪资总额。
以下是示例代码:
Mapper类:
```java
public class SalaryMapper extends Mapper<Object, Text, Text, DoubleWritable> {
private Text name = new Text();
private DoubleWritable salary = new DoubleWritable();
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(",");
name.set(fields[0]);
salary.set(Double.parseDouble(fields[1]));
context.write(name, salary);
}
}
```
Reducer类:
```java
public class SalaryReducer extends Reducer<Text, DoubleWritable, Text, DoubleWritable> {
private DoubleWritable totalSalary = new DoubleWritable();
public void reduce(Text key, Iterable<DoubleWritable> values, Context context) throws IOException, InterruptedException {
double sum = 0;
for (DoubleWritable val : values) {
sum += val.get();
}
totalSalary.set(sum);
context.write(key, totalSalary);
}
}
```
最后,在main函数中设置Job的相关参数,并且指定输入输出路径。调用Job的waitForCompletion方法提交任务。
```java
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "salary count");
job.setJarByClass(SalaryCount.class);
job.setMapperClass(SalaryMapper.class);
job.setReducerClass(SalaryReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(DoubleWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
```
其中,args[0]是输入文件路径,args[1]是输出文件路径。在IDE环境下可以直接运行程序,而在伪分布式集群上需要将程序打包成jar包,然后使用hadoop jar命令提交任务。
相关推荐
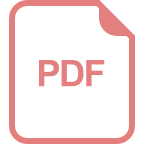
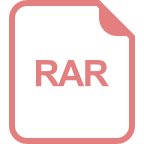














