运用c++定义一个Point类,派生出Rectangle和Circle类,计算个派生类对象的面积Area()。 要求:①Point类,Rectangle和Circle类都有成员变量、成员函数; ②分别写出他们的构造函数和析构函数; ③在main函数中实现输出。 ④力争做到数据输入输出的直观性。
时间: 2024-02-18 14:02:15 浏览: 28
好的,这是一个经典的面向对象编程问题,下面是代码实现:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const double PI = 3.1415926535897932384626;
// Point类
class Point {
protected:
double x, y;
public:
Point(double x = 0, double y = 0) : x(x), y(y) {}
~Point() {}
double getX() const { return x; }
double getY() const { return y; }
void setX(double x) { this->x = x; }
void setY(double y) { this->y = y; }
};
// Rectangle类
class Rectangle : public Point {
protected:
double width, height;
public:
Rectangle(double x = 0, double y = 0, double width = 0, double height = 0) : Point(x, y), width(width), height(height) {}
~Rectangle() {}
double getWidth() const { return width; }
double getHeight() const { return height; }
void setWidth(double width) { this->width = width; }
void setHeight(double height) { this->height = height; }
double Area() const { return width * height; }
};
// Circle类
class Circle : public Point {
protected:
double radius;
public:
Circle(double x = 0, double y = 0, double radius = 0) : Point(x, y), radius(radius) {}
~Circle() {}
double getRadius() const { return radius; }
void setRadius(double radius) { this->radius = radius; }
double Area() const { return PI * radius * radius; }
};
int main() {
// 创建对象
Rectangle rect(1, 2, 3, 4);
Circle circle(1, 2, 5);
// 输出面积
cout << "Rectangle area: " << rect.Area() << endl;
cout << "Circle area: " << circle.Area() << endl;
return 0;
}
```
在这个代码中,我们定义了一个`Point`类,表示二维平面上的一个点;然后我们派生出了`Rectangle`和`Circle`类,分别表示矩形和圆形。每个类都有自己的构造函数和析构函数,并且都有一个计算面积的函数`Area()`。在`main()`函数中,我们创建了一个矩形对象和一个圆形对象,并且输出它们的面积。
值得注意的是,我们在`Circle`类中定义了一个常量`PI`,表示圆周率。这是因为C++标准库中并没有定义这个常量,所以我们需要自己定义。在这里,我们使用了C++中的`const`关键字来表示`PI`是一个常量,避免了在程序中不小心修改`PI`的值。
相关推荐
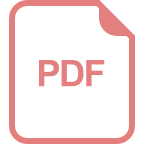
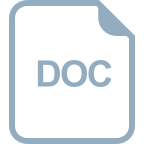
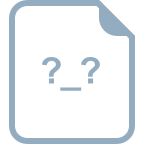










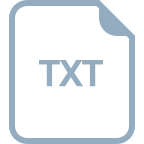
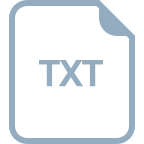
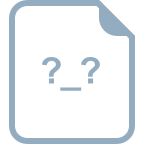