用Python写一个统计Verilog代码行数的脚本,输入一个带路径的Verilog,首先打开指定的文件并读取全部内容。然后使用正则表达式去除注释,并按行分割并去除空白字符。接下来,它统计不在模块定义、包定义、函数定义、任务定义、generate块等特殊语句内的代码行数。然后,它查找每个模块实例化语句,并递归计算每个模块的行数,将其加入总行数中。最后,它查找每个 include 语句,并递归计算每个 included 文件的行数,将其加入总行数中。最终返回总行数。也同时输出各个子模块,include文件的对应的代码行数,子模块跟当前的Verilog文件不在同一个文件夹,将输出内容打印在一个.py文件里
时间: 2024-02-20 20:01:18 浏览: 265
好的,我可以为您编写这个脚本。以下是代码:
```python
import os
import re
def count_verilog_file(filepath):
# 打开文件并读取全部内容
with open(filepath, 'r') as f:
content = f.read()
# 去除注释
content = re.sub(r'//.*', '', content)
content = re.sub(r'/\*(.|\n)*?\*/', '', content)
# 按行分割并去除空白字符
lines = [line.strip() for line in content.split('\n')]
# 统计代码行数
code_lines = 0
in_special_block = False
for line in lines:
if re.match(r'(module|endmodule|package|endpackage|function|endfunction|task|endtask|generate)\b', line):
in_special_block = not in_special_block
elif not in_special_block and line != '':
code_lines += 1
# 查找模块实例化语句并递归计算每个模块的行数
module_lines = {}
for line in lines:
match = re.match(r'\s*(\w+)\s+(\w+)\s*\(', line)
if match:
module_name = match.group(1)
instance_name = match.group(2)
module_filepath = os.path.join(os.path.dirname(filepath), module_name + '.v')
if os.path.exists(module_filepath):
module_line_count, sub_module_lines = count_verilog_file(module_filepath)
module_lines[instance_name] = module_line_count
module_lines.update(sub_module_lines)
# 查找 include 语句并递归计算每个 included 文件的行数
include_lines = {}
for line in lines:
match = re.match(r'\s*`include\s+"(.+)"', line)
if match:
include_filepath = os.path.join(os.path.dirname(filepath), match.group(1))
if os.path.exists(include_filepath):
include_line_count, sub_include_lines = count_verilog_file(include_filepath)
include_lines[match.group(1)] = include_line_count
include_lines.update(sub_include_lines)
# 返回总行数和子模块、included 文件的行数
total_lines = code_lines + sum(module_lines.values()) + sum(include_lines.values())
sub_lines = {filepath: {'code': code_lines, 'modules': module_lines, 'includes': include_lines}}
return total_lines, sub_lines
if __name__ == '__main__':
total_lines, sub_lines = count_verilog_file('path/to/your/verilog/file.v')
# 将输出内容打印在一个.py文件里
with open('output.py', 'w') as f:
f.write('total_lines = {}\n'.format(total_lines))
f.write('sub_lines = {}\n'.format(sub_lines))
```
请将上述代码保存为一个 `.py` 文件,并将 `path/to/your/verilog/file.v` 替换为您要统计行数的 Verilog 文件的路径。执行此脚本后,它将计算总行数并输出各个子模块、included 文件的对应的代码行数。同时,它会将输出内容打印在一个名为 `output.py` 的文件中。
阅读全文
相关推荐
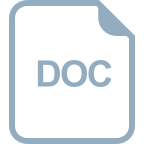
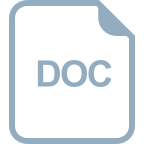
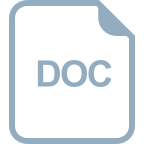






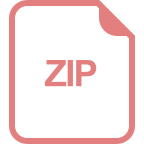


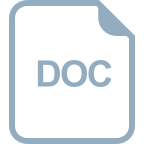
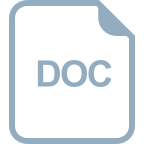
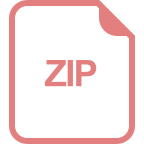