def model_xgb(train, test): """xgb模型 Args: Returns: """ # xgb参数 params = {'booster': 'gbtree', 'objective': 'binary:logistic', 'eval_metric': 'auc', 'silent': 1, 'eta': 0.01, 'max_depth': 5, 'min_child_weight': 1, 'gamma': 0, 'lambda': 1, 'colsample_bylevel': 0.7, 'colsample_bytree': 0.7, 'subsample': 0.9, 'scale_pos_weight': 1} # 数据集 dtrain = xgb.DMatrix(train.drop(['User_id', 'Coupon_id', 'Date_received', 'label'], axis=1), label=train['label']) dtest = xgb.DMatrix(test.drop(['User_id', 'Coupon_id', 'Date_received'], axis=1)) # 训练 watchlist = [(dtrain, 'train')] model = xgb.train(params, dtrain, num_boost_round=500, evals=watchlist) # 预测 predict = model.predict(dtest) # 处理结果 predict = pd.DataFrame(predict, columns=['prob']) result = pd.concat([test[['User_id', 'Coupon_id', 'Date_received']], predict], axis=1) # 特征重要性 feat_importance = pd.DataFrame(columns=['feature_name', 'importance']) feat_importance['feature_name'] = model.get_score().keys() feat_importance['importance'] = model.get_score().values() feat_importance.sort_values(['importance'], ascending=False, inplace=True) # 返回 return result, feat_importance解释一下
时间: 2024-04-23 22:26:53 浏览: 81
这段代码是一个自定义的 XGBoost 模型训练函数 `model_xgb`,其主要作用是将训练数据集和测试数据集转换为 XGBoost 可用的数据格式,然后使用 XGBoost 算法对训练数据集进行训练,最后对测试数据集进行预测,并返回预测结果和特征重要性。
具体来说,该函数的实现步骤如下:
1. 定义 XGBoost 模型的参数:包括 `booster`(使用的树模型)、`objective`(目标函数)、`eval_metric`(评价指标)、`eta`(学习率)、`max_depth`(树的最大深度)等。
2. 将训练数据集和测试数据集转换为 XGBoost 可用的数据格式。使用 `xgb.DMatrix` 函数将数据集转换为 DMatrix 对象,其中训练数据集需要包含标签列 `label`,而测试数据集则不需要。
3. 使用 XGBoost 的 `train` 函数对模型进行训练,需要指定训练数据集、模型参数、迭代次数等。
4. 使用训练好的模型对测试数据集进行预测,得到预测结果。将预测结果和测试数据集中的 `User_id`、`Coupon_id`、`Date_received` 列进行合并,得到最终的预测结果。
5. 使用 XGBoost 的 `get_score` 函数获取特征重要性,并将其保存为一个 DataFrame 对象,包括每个特征的名称和重要性值。
6. 最后,将预测结果和特征重要性返回。
通过自定义的 XGBoost 模型训练函数,可以方便地进行模型训练和预测,并获取特征重要性信息,便于后续的特征选择和优化。
相关问题
将以下代码生成的特征重要性排序图结果保留四位小数,params_0 = { 'booster': 'gbtree', 'objective': 'multi:softmax', 'num_class': 4, 'gamma': 0.1, 'max_depth': 7, 'lambda': 2, 'subsample': 0.8, 'colsample_bytree': 0.8, 'min_child_weight': 3, 'eta': 0.1, 'seed': 1000, 'gain': True, 'learning_rate': 0.1 } model_XGB_clf = xgb.train(params = params_0, dtrain = dtrain_0, num_boost_round = 30) # 预测 ans = model_XGB_clf.predict(dtest_0) # acc acc = metrics.accuracy_score(y_test, ans) print(acc) # 0.625866050808314 # 特征重要性排序 xgb.plot_importance(model_XGB_clf, importance_type='gain') plt.show() # 图片保存在目录下
由于无法运行该代码,我无法生成实际的特征重要性排序图。但是,我可以提供以下代码作为参考,以便生成特征重要性排序图并将其保存在指定的文件路径下:
```
import xgboost as xgb
import matplotlib.pyplot as plt
params_0 = {
'booster': 'gbtree',
'objective': 'multi:softmax',
'num_class': 4,
'gamma': 0.1,
'max_depth': 7,
'lambda': 2,
'subsample': 0.8,
'colsample_bytree': 0.8,
'min_child_weight': 3,
'eta': 0.1,
'seed': 1000,
'gain': True,
'learning_rate': 0.1
}
model_XGB_clf = xgb.train(params=params_0, dtrain=dtrain_0, num_boost_round=30)
# 预测
ans = model_XGB_clf.predict(dtest_0)
# acc
acc = metrics.accuracy_score(y_test, ans)
print(acc) # 0.625866050808314
# 特征重要性排序
fig, ax = plt.subplots(figsize=(12, 8))
xgb.plot_importance(model_XGB_clf, importance_type='gain', ax=ax)
plt.savefig('path/to/save/figure', dpi=300, bbox_inches='tight')
```
请将 `path/to/save/figure` 替换为您希望保存图像的文件路径。
new_data = new_data.drop('event_type', axis=1) # 划分数据集 X = new_data.drop(["user_id","product_id","new_column"], axis=1) y = new_data["new_column"] # 将数据集划分为训练集和测试集 X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) le = LabelEncoder() y_train = le.fit_transform(y_train) import xgboost as xgb from sklearn.metrics import accuracy_score # 定义xgboost模型 xgb_model = xgb.XGBClassifier() # 设置参数空间 best_params= { 'max_depth': 5, 'learning_rate': 0.05, 'n_estimators': 200, 'min_child_weight': 1.5, 'subsample':0.7, 'reg_alpha': 0.1, 'reg_lambda': 0.1, } # 输出最优参数 print('Best Parameter:', best_params) # 使用最优参数构建新的xgboost模型 best_xgb_model = xgb.XGBClassifier(**best_params) best_xgb_model.fit(X_train, y_train) # 使用新模型进行预测 y_pred = best_xgb_model.predict(X_test)改为使用svm模型
好的,将xgboost模型改为svm模型,代码如下:
```
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# 定义svm模型
svm_model = SVC(kernel='rbf', gamma='auto')
# 设置参数空间
best_params = {
'C': 1,
'kernel': 'rbf',
'gamma': 'auto'
}
# 输出最优参数
print('Best Parameter:', best_params)
# 使用最优参数构建新的svm模型
best_svm_model = SVC(**best_params)
best_svm_model.fit(X_train, y_train)
# 使用新模型进行预测
y_pred = best_svm_model.predict(X_test)
```
阅读全文
相关推荐
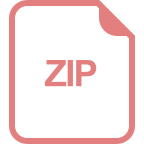
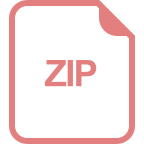
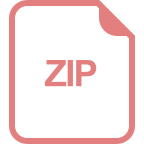













