平衡二叉树的调整c语言代码
时间: 2024-06-13 07:02:01 浏览: 14
平衡二叉树,如AVL树或红黑树,是自平衡的二叉搜索树,确保在插入和删除节点后树的高度始终保持在相对较小的范围内。C语言中实现这类数据结构通常会涉及递归和结构体定义。这里是一个简单的AVL树节点和旋转调整函数的基本框架:
```c
// 定义一个AVL树节点结构
typedef struct AVLNode {
int key; // 节点存储的数据
struct AVLNode *left, *right; // 左右子节点
int height; // 节点高度
} AVLNode;
// 插入节点的函数(包含旋转调整)
AVLNode* insert(AVLNode* root, int key) {
// ...其他插入操作...
// 旋转调整
if (/* 条件判断导致不平衡 */)
{
if (/* 需要左旋的情况 */)
return leftRotate(root);
else if (/* 需要右旋的情况 */)
return rightRotate(root);
}
return root;
}
// 左旋函数
AVLNode* leftRotate(AVLNode* z) {
AVLNode* y = z->right;
AVLNode* T2 = y->left;
// ...旋转后的子节点链接和高度更新...
return y;
}
// 右旋函数
AVLNode* rightRotate(AVLNode* y) {
AVLNode* x = y->left;
AVLNode* T2 = x->right;
// ...旋转后的子节点链接和高度更新...
return x;
}
// 更多辅助函数,如获取高度、检查平衡等
// 示例:
// AVLNode* createAVLTree() { ... } // 初始化空树
// AVLNode* root = createAVLTree();
// root = insert(root, value);
```
相关推荐
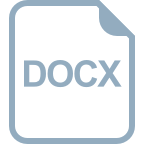
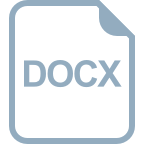











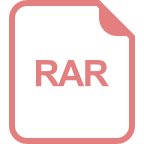
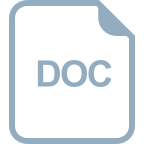
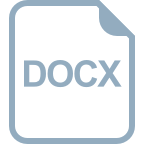
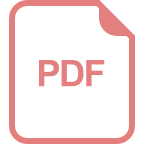