#!/bin/sh #HOME=/ BIN=PLCXPro stoprun() { PID=`ps -ef --cols=200|grep -v stopapp |grep "$1" | awk '{print $2 " " $8}'|sed -e '/grep/d' -e 's/^ *//' -e 's/ .*//'` if [ -n "$PID" ] then echo "$1 id is $PID, kill normal process!" kill -3 $PID else echo "$1 id $PID is down! it's over!" fi } stoprunstart() { PID=`ps -ef --cols=200|grep -v stopapp |grep "$1"|grep start | awk '{print $2 " " $8}'|sed -e '/grep/d' -e 's/^ *//' -e 's/ .*//'` if [ -n "$PID" ] then echo "$1 id is $PID, kill normal process!" kill -9 $PID else echo "$1 id $PID is down! it's over!" fi } runtest() { PID=`ps -ef --cols=200|grep -v stopapp |grep "$1" | awk '{print $2 " " $8}'|sed -e '/grep/d' -e 's/^ *//' -e 's/ .*//'` if [ -n "$PID" ] then ## echo $1 id is $PID, sys normal process! sleep 30 else echo $1 is down! reload the process! date echo nohup $BIN & nohup ./$BIN > /dev/null & fi }怎么开启或者重启这个PLCXPro
时间: 2024-03-05 08:52:53 浏览: 25
可以运行以下命令来重启 PLCXPro 进程:
```
./stopapp.sh PLCXPro
```
该命令会执行 stoprun 函数,查找并终止名为 PLCXPro 的进程。
然后再执行以下命令来启动 PLCXPro 进程:
```
./runapp.sh PLCXPro
```
该命令会执行 runtest 函数,查找名为 PLCXPro 的进程是否已经存在,若不存在则启动 PLCXPro 进程。
相关问题
pad = (num_cols - num_low_freqs + 1) // 2
这是一个关于 Python 语言的问题,pad 是一个变量,表示填充的数量,num_cols 和 num_low_freqs 是整数变量, // 表示整除运算符,1 是常数。根据公式,pad 的值等于 (num_cols - num_low_freqs + 1) 整除 2 的结果。
[rows,cols] = find(P1==-1); [rows,cols]
这段代码使用 `find` 函数来查找矩阵 `P1` 中所有值为 -1 的元素的行索引和列索引。下面是代码的完整示例:
```matlab
[rows, cols] = find(P1 == -1);
```
`find(P1 == -1)` 返回一个列向量,其中每个元素对应一个值为 -1 的元素在矩阵 `P1` 中的位置。然后,`[rows, cols]` 将这个列向量分解为行索引和列索引,存储在 `rows` 和 `cols` 中。
你可以使用 `rows` 和 `cols` 来访问矩阵 `P1` 中所有值为 -1 的元素的位置。例如,可以通过以下方式打印出这些位置:
```matlab
for i = 1:length(rows)
fprintf('Element -1 found at position (%d, %d)\n', rows(i), cols(i));
end
```
这将逐行打印出所有值为 -1 的元素在矩阵 `P1` 中的位置。
相关推荐
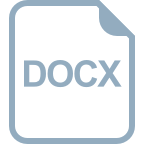
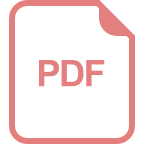
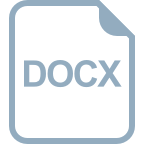













