org.bouncycastle.util.encoders.DecoderException: unable to decode base64 string: String index out of range: -4
时间: 2024-04-25 08:24:40 浏览: 249
这个错误是由于尝试解码一个无效的 base64 字符串而引起的。具体说来,这个错误是因为字符串的索引超出范围导致的。你可以检查一下你提供的 base64 字符串是否正确,并确保没有出现任何错误或截断。另外,你也可以尝试使用其他的 base64 解码方法来处理这个字符串。如果问题仍然存在,可能需要进一步检查代码逻辑或调整输入数据来解决问题。
相关问题
org.bouncycastle.util.encoders.DecoderException: exception decoding Hex string: invalid characters encountered in Hex string
This exception is thrown when trying to decode a hexadecimal string that contains invalid characters. Hexadecimal strings should only contain characters from 0-9 and A-F (or a-f). If the string contains any other characters, such as spaces or special characters, the decoding process fails and this exception is thrown.
To fix this issue, check the hexadecimal string and make sure it only contains valid characters. If necessary, remove any invalid characters before attempting to decode the string.
BouncyCastle使用
Bouncy Castle可以通过下载相应的jar包并将其添加到项目中来使用。在Java中,你可以使用Bouncy Castle提供的API来访问其提供的加密算法和协议。下面是一个简单的示例,演示如何使用Bouncy Castle进行AES加密和解密:
```java
import org.bouncycastle.crypto.BufferedBlockCipher;
import org.bouncycastle.crypto.engines.AESEngine;
import org.bouncycastle.crypto.modes.CBCBlockCipher;
import org.bouncycastle.crypto.paddings.PaddedBufferedBlockCipher;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithIV;
import org.bouncycastle.util.encoders.Base64;
import java.security.SecureRandom;
public class BouncyCastleExample {
private static final int KEY_SIZE = 256;
private static final int IV_SIZE = 128;
private static final int BLOCK_SIZE = 128;
private static final String KEY = "my_secret_key";
private static final String PLAIN_TEXT = "Hello, world!";
public static void main(String[] args) throws Exception {
SecureRandom random = new SecureRandom();
byte[] keyBytes = KEY.getBytes();
byte[] ivBytes = new byte[IV_SIZE / 8];
random.nextBytes(ivBytes);
BufferedBlockCipher cipher = new PaddedBufferedBlockCipher(new CBCBlockCipher(new AESEngine()));
KeyParameter keyParam = new KeyParameter(keyBytes);
ParametersWithIV params = new ParametersWithIV(keyParam, ivBytes);
cipher.init(true, params);
byte[] input = PLAIN_TEXT.getBytes();
byte[] output = new byte[cipher.getOutputSize(input.length)];
int len = cipher.processBytes(input, 0, input.length, output, 0);
len += cipher.doFinal(output, len);
String base64Encoded = new String(Base64.encode(output));
System.out.println("Encrypted text: " + base64Encoded);
cipher = new PaddedBufferedBlockCipher(new CBCBlockCipher(new AESEngine()));
params = new ParametersWithIV(keyParam, ivBytes);
cipher.init(false, params);
byte[] decoded = Base64.decode(base64Encoded);
byte[] decrypted = new byte[cipher.getOutputSize(decoded.length)];
len = cipher.processBytes(decoded, 0, decoded.length, decrypted, 0);
len += cipher.doFinal(decrypted, len);
System.out.println("Decrypted text: " + new String(decrypted));
}
}
```
在上面的示例中,我们使用Bouncy Castle提供的AES算法和CBC模式来加密和解密数据。我们首先生成一个随机的IV(初始化向量),并使用它和我们的密钥一起初始化加密/解密器。在加密时,我们将输入数据转换为字节数组,将其传递给加密器,并将加密后的结果编码为Base64字符串。在解密时,我们对编码后的字符串进行解码,然后使用相同的IV和密钥初始化解密器,并将解密后的结果转换为字符串。
阅读全文
相关推荐
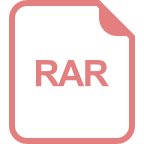
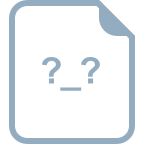
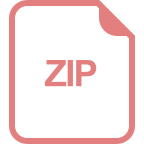
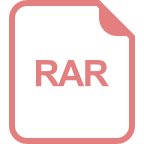
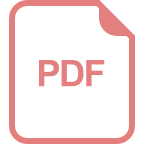
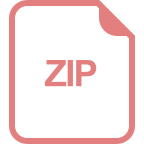
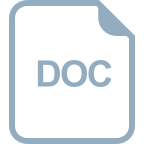
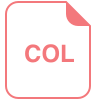






