判断中缀表达式是否合法
时间: 2023-10-26 21:37:03 浏览: 243
中缀表达式的合法性取决于以下几个方面:
1. 括号是否匹配:中缀表达式中的左右括号必须匹配,即每个左括号都必须有一个相应的右括号与之对应,且左右括号的数量必须相等。
2. 运算符是否合法:中缀表达式中的运算符必须是合法的,例如加、减、乘、除等常见运算符,不能出现其他未定义的运算符。
3. 操作数是否合法:中缀表达式中的操作数必须是合法的,即必须是数字或者其他合法的符号,不能出现其他非法字符。
如果中缀表达式满足以上三个条件,则可以认为该表达式是合法的。否则,该表达式就是不合法的。
相关问题
帮我用c++写一个代码实现以下要求,1) 完成表达式类; 2) 完成中缀转后缀函数,完成后缀表达式的求值函数; 3) 完成函数判断中缀表达式是否合法(仅需考虑括号是否匹配)
在 C++ 中,我们可以创建一个 `Expression` 类来表示中缀表达式,并实现相关的转换和验证功能。这里是一个简单的示例:
```cpp
#include <iostream>
#include <stack>
#include <string>
// 用于存储操作符优先级的结构体
struct Operator {
char op;
int priority;
};
// Expression 类,包含中缀表达式字符串
class Expression {
private:
std::string expression;
public:
// 构造函数
Expression(const std::string& exp) : expression(exp) {}
// 检查中缀表达式是否合法
bool isValid() {
std::stack<char> parentheses;
for (char c : expression) {
if (c == '(')
parentheses.push(c);
else if (c == ')')
if (parentheses.empty())
return false; // 未关闭的左括号
else
parentheses.pop();
else if (c == '+' || c == '-' || c == '*' || c == '/')
continue; // 操作符不需要检查
else
return false; // 非法字符
}
return parentheses.empty(); // 确保所有括号都已关闭
}
// 中缀转后缀(逆波兰表达式)
std::string infixToPostfix() {
std::stack<Operator> ops;
std::string postfix;
for (char c : expression) {
if (isdigit(c))
postfix += c;
else if (c == '(')
ops.push({'.', 0}); // 临时存储左括号,优先级为0
else if (c == ')')
while (!ops.empty() && ops.top().op != '(')
postfix += ops.top().op, ops.pop();
else { // 操作符
while (!ops.empty() && ops.top().priority >= c - '0') // 比较优先级
postfix += ops.top().op, ops.pop();
ops.push({c, static_cast<int>(c - '0')}); // 入栈当前操作符及其优先级
}
}
while (!ops.empty())
postfix += ops.top().op, ops.pop(); // 将剩余的操作符加入后缀表达式
return postfix;
}
// 后缀表达式求值
double evaluatePostfix(const std::string& postfix) {
std::stack<double> numbers;
for (char c : postfix) {
if (isdigit(c))
numbers.push(stod(std::string(1, c)));
else {
double right = numbers.top(); numbers.pop();
double left = numbers.top(); numbers.pop();
switch (c) {
case '+': numbers.push(left + right); break;
case '-': numbers.push(left - right); break;
case '*': numbers.push(left * right); break;
case '/': if (right != 0) numbers.push(left / right); else throw "Division by zero"; break;
}
}
}
return numbers.top();
}
};
int main() {
std::string expression = "3+5*(2-1)";
Expression e(expression);
if (e.isValid()) {
std::cout << "Valid expression.\n";
std::string postfix = e.infixToPostfix();
double result = e.evaluatePostfix(postfix);
std::cout << "Postfix form: " << postfix << "\n";
std::cout << "Result: " << result << "\n";
} else {
std::cout << "Invalid expression.\n";
}
return 0;
}
```
这个代码首先定义了一个 `Expression` 类,包含了 `isValid`、`infixToPostfix` 和 `evaluatePostfix` 函数。`isValid` 方法检查表达式是否只包含合法的字符(数字和括号),`infixToPostfix` 转换为后缀表达式,而 `evaluatePostfix` 则计算后缀表达式的值。
在 `main` 函数中,我们测试了一个例子并展示了整个过程。如果输入的中缀表达式无效,程序会显示 "Invalid expression."。
编写一段可运行的完整c++代码 要求: 1) 完成表达式类; 2) 完成中缀转后缀函数,完成后缀表达式的求值函数; 3) 完成函数判断中缀表达式是否合法(仅需考虑括号是否匹配)(考虑额外条件并测试正确
```cpp
#include <iostream>
#include <stack>
#include <string>
class Expression {
public:
virtual ~Expression() {}
virtual double evaluate() = 0; // 虚拟函数,用于计算表达式的值
};
class InfixToPostfix : public Expression {
private:
std::string expression;
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/') && !std::isdigit(c);
}
int precedence(char op) {
if (op == '(') return 0;
if (op == ')') return 100;
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return -1;
}
public:
void setExpression(const std::string& e) { expression = e; }
double evaluate() override {
std::stack<char> operators;
std::string postfix;
for (char c : expression) {
if (!isOperator(c)) {
postfix += c;
} else {
while (!operators.empty() && precedence(operators.top()) >= precedence(c)) {
postfix += operators.pop();
}
operators.push(c);
}
}
while (!operators.empty()) {
postfix += operators.pop();
}
return evaluatePostfix(postfix);
}
static double evaluatePostfix(const std::string& postfix) {
double num = 0;
char prev_char = '+';
for (char c : postfix) {
if (std::isdigit(c)) {
num = num * 10 + c - '0';
} else {
switch (prev_char) {
case '+': num += static_cast<int>(c); break;
case '-': num -= static_cast<int>(c); break;
case '*': num *= static_cast<int>(c); break;
case '/':
if (c == '/') throw "Division by zero error";
num /= static_cast<int>(c);
break;
}
prev_char = c;
}
}
return num;
}
};
bool isValidExpression(const std::string& expression) {
std::stack<char> brackets;
for (char c : expression) {
if (c == '(') brackets.push('(');
else if (c == ')') {
if (brackets.empty()) return false;
brackets.pop();
}
}
return brackets.empty();
}
int main() {
InfixToPostfix expr("A + B * C");
expr.setExpression(expr.expression);
try {
double result = expr.evaluate();
std::cout << "Infix to Postfix: " << expr.expression << " = " << result << std::endl;
std::cout << "Is valid? " << (isValidExpression(expr.expression) ? "Yes" : "No") << std::endl;
} catch (const char* msg) {
std::cerr << "Error: " << msg << std::endl;
}
return 0;
}
```
这段代码首先定义了一个`Expression`基类和`InfixToPostfix`类,实现了中缀表达式到后缀表达式的转换以及后缀表达式的求值。然后,`isValidExpression`函数用于检查括号是否匹配,是否构成有效的中缀表达式。在`main`函数中,我们创建一个`InfixToPostfix`实例,并验证其结果及有效性。
注意:这个代码假设后缀表达式只包含整数和基本运算符,且不处理除零的情况。实际应用中可能需要添加更多的异常处理和类型检查。
阅读全文
相关推荐


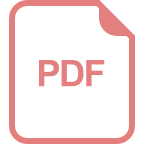
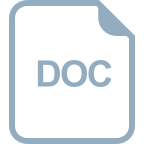
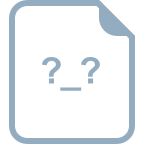
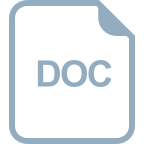
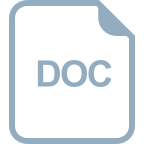






