如何用Java语言实现中缀表达式合理性判断
时间: 2024-03-02 14:51:05 浏览: 196
在Java中,可以使用栈(Stack)来实现中缀表达式的合理性判断。具体实现步骤如下:
1. 创建一个栈(Stack);
2. 遍历中缀表达式中的每个字符,如果该字符是左括号,则将其压入栈中;
3. 如果该字符是右括号,则弹出栈顶元素,如果栈顶元素不是左括号,则表达式不合法;
4. 如果该字符是操作符,则弹出栈顶元素,如果栈顶元素不是左括号,则表达式不合法;否则将该操作符压入栈中;
5. 如果该字符是数字或变量,则将其压入栈中;
6. 遍历完中缀表达式后,如果栈中还有元素,则表达式不合法;
7. 如果栈中没有元素,则表达式合法。
下面是一个示例代码:
```java
import java.util.Stack;
public class InfixExpressionValidity {
public static boolean isValid(String infix) {
Stack<Character> stack = new Stack<>();
for (int i = 0; i < infix.length(); i++) {
char ch = infix.charAt(i);
if (ch == '(') {
stack.push(ch);
} else if (ch == ')') {
if (stack.isEmpty() || stack.pop() != '(') {
return false;
}
} else if (isOperator(ch)) {
if (stack.isEmpty() || stack.peek() == '(') {
return false;
}
stack.push(ch);
} else if (isOperand(ch)) {
stack.push(ch);
}
}
return stack.isEmpty();
}
private static boolean isOperator(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
private static boolean isOperand(char ch) {
return Character.isDigit(ch) || Character.isLetter(ch);
}
public static void main(String[] args) {
String infix = "1+2*(3-4)/5";
boolean isValid = isValid(infix);
System.out.println("Infix expression " + infix + " is " + (isValid ? "valid" : "invalid"));
}
}
```
该程序可以判断中缀表达式 `1+2*(3-4)/5` 的合理性,输出结果为:
```
Infix expression 1+2*(3-4)/5 is valid
```
阅读全文
相关推荐
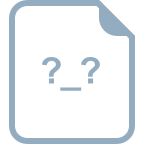
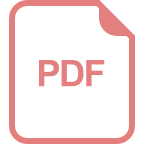
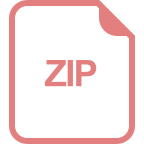
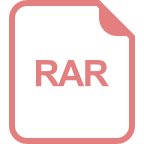
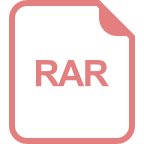
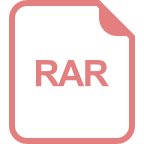
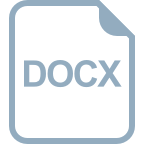
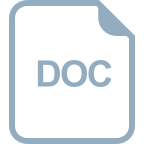
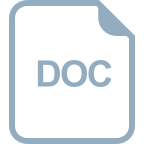
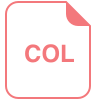
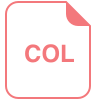
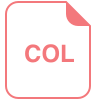
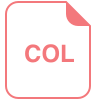
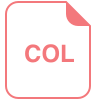
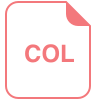
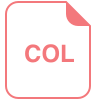
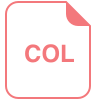
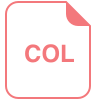