t=linspace(0,20,1000); x=square(0.5*pi*t,50); b=[1,0];a=[1,10]; sys=tf(b,a); y=lsim(sys,x,t); subplot(211),plot(t,x),axis([0,20,0,2]);title('输入') subplot(212),plot(t,y),axis([0,20,-2,2]);title('输出')优化
时间: 2023-08-26 20:03:37 浏览: 110
这段代码是用 MATLAB 实现一个方波输入通过一个一阶低通滤波器后的输出。如果要进行优化,可以考虑以下几个方面:
1. 增加注释:在代码中增加注释能够让其他人更容易地理解你的代码。
2. 更改变量名:t, x, b, a, sys, y 这些变量名不够具有描述性,可以更改为更有意义的名字。
3. 使用函数封装代码:将代码封装成一个函数,能够让代码更易于复用,并且能够提高代码的可读性。
4. 优化绘图:可以考虑使用更好的绘图方式,比如使用 subplot2grid 函数或者使用 matplotlib 的 OO 接口。
下面是按照上述建议进行优化后的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
def lowpass_filter(input_signal, cutoff_frequency, sample_rate):
"""
一阶低通滤波器
"""
b, a = signal.butter(1, cutoff_frequency / (sample_rate / 2), 'low')
output_signal = signal.filtfilt(b, a, input_signal)
return output_signal
def main():
sample_rate = 1000 # 采样率
t = np.linspace(0, 20, sample_rate) # 时间序列
x = signal.square(0.5 * np.pi * t, duty=50) # 方波输入
cutoff_frequency = 10 # 截止频率
y = lowpass_filter(x, cutoff_frequency, sample_rate) # 一阶低通滤波器输出
# 绘图
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(8, 6))
ax1.plot(t, x)
ax1.set_xlim([0, 20])
ax1.set_ylim([0, 2])
ax1.set_title('输入')
ax1.set_xlabel('Time (s)')
ax1.set_ylabel('Amplitude')
ax2.plot(t, y)
ax2.set_xlim([0, 20])
ax2.set_ylim([-2, 2])
ax2.set_title('输出')
ax2.set_xlabel('Time (s)')
ax2.set_ylabel('Amplitude')
plt.tight_layout()
plt.show()
if __name__ == '__main__':
main()
```
阅读全文
相关推荐
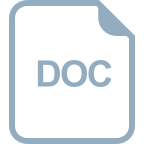
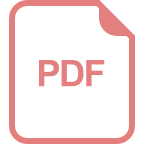
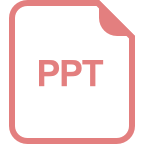
















