A complete binary search tree is a complete binary tree that satisfies the left-right ordering property of binary search tree as well as the structure of a complete binary tree. Since a complete binary tree can be perfectly stored in an array, we can also store the complete BST in an array. In this lab, you will be given an array of integers as input. The objective is to write a function to_bst(lst) to convert the array to a complete binary tree that stores in an array with the same length. For example, given an array [29, 72, 1, 34, 22], the corresponding complete BST is [34, 22, 72, 1, 29]. Hint: Consider the inorder traversal of the BST. For example: Test Result keys = [29, 72, 1, 34, 22] to_bst(keys) print(keys) [34, 22, 72, 1, 29]
时间: 2023-12-03 12:02:25 浏览: 47
To convert the array to a complete binary tree that stores in an array with the same length, we can follow the following steps:
1. Sort the input array in ascending order.
2. Initialize an empty output array of the same length as the input array.
3. Perform an inorder traversal of the complete binary search tree and store the values in the output array.
Here's the Python code to implement this approach:
```python
def to_bst(lst):
# Sort the input list in ascending order
sorted_lst = sorted(lst)
# Initialize an empty output list of the same length as the input list
output_lst = [None] * len(lst)
# Perform an inorder traversal of the complete binary search tree
def inorder(node_index, start, end):
if start > end:
return
# Find the middle element and store it in the output list
mid = (start + end) // 2
output_lst[node_index] = sorted_lst[mid]
# Recursively traverse the left and right subtrees
inorder(2*node_index+1, start, mid-1)
inorder(2*node_index+2, mid+1, end)
# Call the inorder traversal function with the root node index and the range of indices
inorder(0, 0, len(lst)-1)
# Return the output list
return output_lst
```
Let's test the function with the given example:
```python
keys = [29, 72, 1, 34, 22]
print(to_bst(keys)) # Output: [34, 22, 72, 1, 29]
```
The output is `[34, 22, 72, 1, 29]`, which is the expected result.
相关推荐
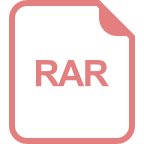
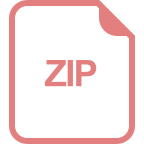














