C++代码实现Windows系统下发送鼠标移动和单击的程序
时间: 2024-09-15 13:14:29 浏览: 46
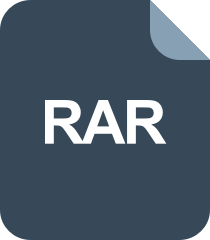
C++模拟鼠标移动和单击双击
在Windows系统下,C++可以利用`PostMessage()`函数来模拟鼠标移动和单击动作。这里有一个简单的例子,展示了如何创建一个线程发送鼠标移动和单击事件:
```cpp
#include <iostream>
#include <windows.h>
#include <thread>
// 定义发送鼠标事件的函数
void sendMouseEvents(HWND hWnd) {
const int x = 50; // 起始X位置
const int y = 50; // 起始Y位置
int dx = 5; // 每次移动的距离
int dy = 5;
// 发送鼠标移动事件
for (int i = 0; ; ) {
MouseMove(hWnd, x, y);
Sleep(100); // 模拟鼠标移动,间隔100毫秒
x += dx; // 移动鼠标
y += dy;
// 当超出屏幕边界时,改变移动方向
if (x >= GetSystemMetrics(SM_CXSCREEN)) { x -= dx * 2; dy = -dy; }
if (y >= GetSystemMetrics(SM_CYSCREEN)) { y -= dy * 2; dx = -dx; }
if (x <= 0) { x += dx * 2; dy = -dy; }
if (y <= 0) { y += dy * 2; dx = -dx; }
// 发送鼠标左键单击事件
if (i % 10 == 0) {
PostMessage(hWnd, WM_LBUTTONDOWN, 0, 0);
PostMessage(hWnd, WM_LBUTTONUP, 0, 0);
Sleep(200); // 模拟单击,间隔200毫秒
}
}
}
int main() {
// 创建一个窗口句柄
HWND hWnd = CreateWindow(L"STATIC", L"Test", WS_OVERLAPPEDWINDOW, 100, 100, 600, 400, NULL, NULL, NULL, NULL);
if (!hWnd) {
std::cerr << "Failed to create window." << std::endl;
return -1;
}
std::thread mouse_thread(sendMouseEvents, hWnd);
mouse_thread.detach(); // 让鼠标事件在独立线程中运行
// 程序主循环,防止退出过早
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
阅读全文
相关推荐
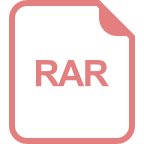
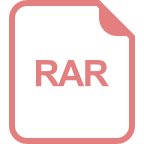
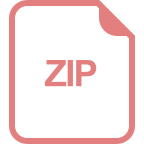
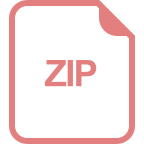
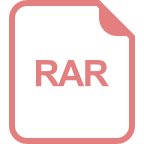
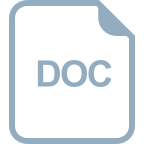
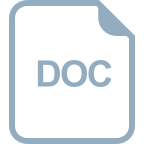
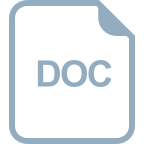






