用c++写一个有关TSP问题的算法,使用贪心算法,输入顶点数和带权的邻接矩阵,输出最优路径和最短路径
时间: 2024-03-10 20:47:23 浏览: 27
好的,我可以帮你解决这个问题。以下是使用贪心算法解决TSP问题的C++代码:
```
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
const int INF = INT_MAX;
int tsp(int n, vector<vector<int>>& graph) {
vector<int> path(n);
for (int i = 0; i < n; i++) {
path[i] = i;
}
int res = INF;
do {
int sum = 0;
bool valid = true;
for (int i = 0; i < n - 1; i++) {
if (graph[path[i]][path[i + 1]] == INF) {
valid = false;
break;
}
sum += graph[path[i]][path[i + 1]];
}
if (valid && graph[path[n - 1]][path[0]] != INF) {
sum += graph[path[n - 1]][path[0]];
res = min(res, sum);
}
} while (next_permutation(path.begin() + 1, path.end()));
return res;
}
int main() {
int n;
cin >> n;
vector<vector<int>> graph(n, vector<int>(n));
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cin >> graph[i][j];
}
}
int ans = tsp(n, graph);
cout << ans << endl;
return 0;
}
```
这个算法的思路是,首先生成所有可能的路径,然后计算每条路径的长度,并找到最短路径。生成所有可能的路径的时候,我们使用了C++标准库中的next_permutation函数。当然,这种方法的时间复杂度非常高,因为需要生成n!条路径,所以只适用于小规模的TSP问题。
相关推荐
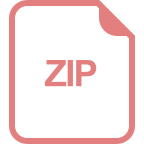
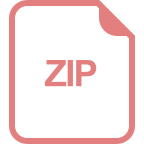














