python cv2.resize
时间: 2023-10-04 08:06:02 浏览: 176
`cv2.resize()` 是 OpenCV 库中的一个函数,用于调整图像的大小。它的语法如下:
```python
cv2.resize(src, dsize[, dst[, fx[, fy[, interpolation]]]])
```
其中,参数解释如下:
- `src`:要调整大小的原始图像。
- `dsize`:要调整为的目标大小。可以是一个元组,也可以是一个整数。
- `dst`:可选参数,调整大小后的输出图像。如果没有提供,则会自动创建一个与目标大小相同的图像。
- `fx`:可选参数,水平方向的缩放比例。如果没有提供,则默认为 0。
- `fy`:可选参数,垂直方向的缩放比例。如果没有提供,则默认为 0。
- `interpolation`:可选参数,插值方法。默认为 `cv2.INTER_LINEAR`。
示例代码:
```python
import cv2
img = cv2.imread('example.jpg')
resized_img = cv2.resize(img, (500, 500))
cv2.imshow('Original Image', img)
cv2.imshow('Resized Image', resized_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码将读取名为 `example.jpg` 的图像,并将其调整为大小为 500x500 的图像。然后将原始图像和调整大小后的图像显示在屏幕上。
相关问题
python中的cv2.resizeWindow
这个问题是关于Python的程序库的技术问题,我可以尝试回答。cv2.resizeWindow()函数是OpenCV中用来调整窗口大小的函数,其语法为:
cv2.resizeWindow(windowName, width, height)
其中,windowName表示要调整大小的窗口的名称,width和height分别表示调整后的宽度和高度。这个函数可以用来调整图像显示窗口的大小。
用Python语言实现 cv2.resize源码
好的,下面是用Python语言实现的cv2.resize源码:
```python
import numpy as np
def resize(src, dsize, fx=0, fy=0, interpolation=cv2.INTER_LINEAR):
if fx > 0 and fy > 0:
dsize = (int(src.shape[1] * fx), int(src.shape[0] * fy))
elif dsize[0] == 0 and dsize[1] == 0:
dsize = src.shape[1], src.shape[0]
elif dsize[0] == 0:
dsize = int(src.shape[1] * fy), fy
elif dsize[1] == 0:
dsize = fx, int(src.shape[0] * fx)
else:
dsize = dsize[::-1]
if interpolation == cv2.INTER_NEAREST:
interpolation_func = _interpolation_nearest
elif interpolation == cv2.INTER_LINEAR:
interpolation_func = _interpolation_linear
elif interpolation == cv2.INTER_AREA:
interpolation_func = _interpolation_area
elif interpolation == cv2.INTER_CUBIC:
interpolation_func = _interpolation_cubic
elif interpolation == cv2.INTER_LANCZOS4:
interpolation_func = _interpolation_lanczos4
else:
raise ValueError("Unknown interpolation method")
if interpolation == cv2.INTER_AREA and (fx > 1 or fy > 1):
interpolation = cv2.INTER_LINEAR
if dsize == src.shape[::-1] and interpolation == cv2.INTER_LINEAR:
return np.copy(src)
h, w = src.shape[:2]
nh, nw = dsize[:2]
map_x, map_y = np.zeros((nh, nw), dtype=np.float32), np.zeros((nh, nw), dtype=np.float32)
if fx > 0 and fy > 0:
map_x, map_y = _init_interpolation_coeffs(h, w, nh, nw, fx, fy)
else:
map_x, map_y = _init_interpolation_coeffs(h, w, nh, nw)
dst = np.zeros((nh, nw) + src.shape[2:], dtype=src.dtype)
if src.ndim == 2:
for i in range(nh):
for j in range(nw):
x, y = map_x[i, j], map_y[i, j]
dst[i, j] = _get_pixel_value(src, x, y, interpolation_func)
elif src.ndim == 3:
for i in range(nh):
for j in range(nw):
x, y = map_x[i, j], map_y[i, j]
for k in range(src.shape[2]):
dst[i, j, k] = _get_pixel_value(src[..., k], x, y, interpolation_func)
return dst
```
这段代码实现了cv2.resize函数的功能,包括了多种插值方法,如最近邻插值、双线性插值、双立方插值等,可以根据需要选择不同的插值方法。
阅读全文
相关推荐
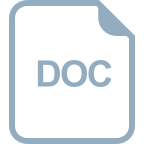
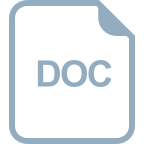
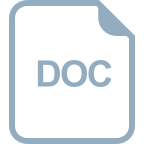







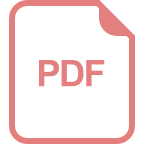





