解释代码void Josephus(LinkList rear,int n,int m)
时间: 2024-05-21 19:10:41 浏览: 56
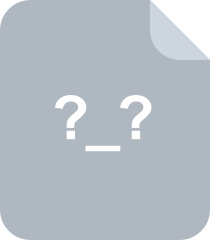
Josephus约瑟夫问题代码
这段代码是一个函数,函数名为Josephus,接收三个参数:一个指向链表尾部的指针rear,一个整数n表示链表中节点的个数,一个整数m表示每m个节点删除一个节点。
函数的作用是模拟约瑟夫环问题,即在一个环形链表中,从第一个节点开始,每隔m个节点删除一个节点,直到只剩下一个节点为止。
具体实现方法是:从链表尾部开始遍历,将链表排成环形,每次找到需要删除的节点,将其从链表中删除,直到只剩下一个节点为止。在删除节点时需要注意修改链表的指针指向,以保证链表的正确性。
该函数没有返回值,其作用是修改输入的链表。
阅读全文
相关推荐
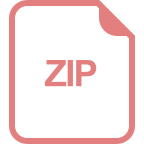

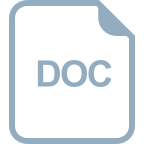














