用Java写一个假设在周末舞会上,男士们和女士们进入舞厅时,各自排成一队。跳舞开始时,依次从男队和女队的队头上各出一人配成舞伴。规定每个舞曲能有一对跳舞者。若两队初始人数不相同,则较长的那一队中未配对者等待下一轮舞曲。现要求写一个程序
时间: 2024-11-16 22:19:46 浏览: 22
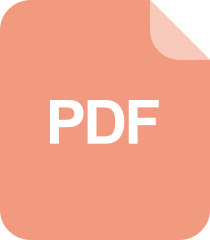
与mp3对应职称英语高频词汇第一部分(带音标)
在Java中,我们可以创建一个简单的模拟程序来实现这个场景。首先,我们定义两个Person类代表男士和女士,包含姓名属性和一个列表来表示队伍。然后,我们可以创建一个舞蹈伴侣选择函数,模拟匹配过程。
```java
import java.util.LinkedList;
import java.util.Queue;
class Person {
String name;
Queue<Person> team;
public Person(String name) {
this.name = name;
this.team = new LinkedList<>();
}
}
public class DanceParty {
private Person[] men; // 男士数组
private Person[] women; // 女士数组
public DanceParty(int[] menSize, int[] womenSize) {
men = new Person[menSize.length];
women = new Person[womenSize.length];
for (int i = 0; i < menSize.length; i++) {
men[i] = new Person("Mr. " + i);
if (menSize[i] > 0) {
men[i].team.add(new Person("Ms. " + i));
}
}
for (int i = 0; i < womenSize.length; i++) {
women[i] = new Person("Ms. " + i);
if (womenSize[i] > 0) {
women[i].team.add(new Person("Mr. " + i));
}
}
}
public void dance() {
while (!isAllPaired()) {
if (men[0].team.isEmpty()) { // 如果男士队列为空,等待女士跳完
System.out.println(men[0].name + " is waiting for a partner.");
} else if (women[0].team.isEmpty()) { // 否则,如果女士队列也空,男士先跳
coupleMenAndWomen(0, 0);
} else { // 否则,谁的队列短就先配对
int shortestQueue = Math.min(men[0].team.size(), women[0].team.size());
coupleMenAndWomen(0, shortestQueue - 1);
}
}
System.out.println("Dancing complete!");
}
private boolean isAllPaired() {
return men[0].team.isEmpty() && women[0].team.isEmpty();
}
private void coupleMenAndWomen(int manIndex, int womanIndex) {
Person man = men[manIndex];
Person woman = women[womanIndex];
man.team.poll(); // 男士移除舞伴
woman.team.poll(); // 女士移除舞伴
System.out.println(man.name + " and " + woman.name + " are dancing together.");
}
}
// 示例用法
public static void main(String[] args) {
int[] menSizes = {5, 3}; // 男士队伍大小
int[] womenSizes = {4, 2}; // 女士队伍大小
DanceParty party = new DanceParty(menSizes, womenSizes);
party.dance();
}
```
在这个程序中,`dance()`方法会持续匹配直到所有舞伴都被配对。`coupleMenAndWomen()`方法负责实际的配对操作。注意,这里假设输入的男士和女士队列长度都是已知的,并且跳舞是按顺序进行的。
阅读全文
相关推荐
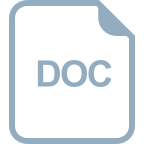
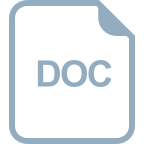















