1:建立一棵二叉树。对此树进行前序遍历、中序遍历及后序遍历,输出遍历序列。(假设序列为:ABD##E#H##CF##G##)
时间: 2024-01-02 09:20:34 浏览: 46
以下是对序列ABD##E#H##CF##G##建立的二叉树的前序遍历、中序遍历和后序遍历:
前序遍历:ABDEHCFG
中序遍历:DBHEAFCG
后序遍历:DHBEFGCA
建立二叉树的过程如下:
1. 将A作为根节点。
2. 将B作为A的左子节点。
3. 将D作为B的左子节点。
4. B没有右子节点,返回A。
5. 将E作为A的右子节点。
6. 将H作为E的左子节点。
7. E没有右子节点,返回A。
8. 将C作为A的右子节点。
9. 将F作为C的左子节点。
10. 将G作为F的右子节点。
11. C没有右子节点,返回A。
相关问题
建立一棵二叉树。对此树进行前序遍历、中序遍历及后序遍历,输出遍历序列。(假设序列为:ABD##E#H##CF##G##)请使用java回答
以下是使用Java实现二叉树的建立和三种遍历方式的代码:
```java
class TreeNode {
char val;
TreeNode left;
TreeNode right;
TreeNode(char x) {
val = x;
}
}
public class BinaryTree {
int index = 0;
public TreeNode buildTree(char[] arr) {
if (arr == null || arr.length == 0) {
return null;
}
return build(arr);
}
private TreeNode build(char[] arr) {
if (index >= arr.length) {
return null;
}
if (arr[index] == '#') {
index++;
return null;
}
TreeNode root = new TreeNode(arr[index]);
index++;
root.left = build(arr);
root.right = build(arr);
return root;
}
public void preOrder(TreeNode root) {
if (root == null) {
return;
}
System.out.print(root.val + " "); preOrder(root.left);
preOrder(root.right); }
public void inOrder(TreeNode root) {
if (root == null) {
return;
}
inOrder(root.left);
System.out.print(root.val + " ");
inOrder(root.right);
}
public void postOrder(TreeNode root) {
if (root == null) {
return;
}
postOrder(root.left);
postOrder(root.right);
System.out.print(root.val + " ");
}
public static void main(String[] args) {
char[] arr = {'A', 'B', 'D', '#', '#', 'E', '#', 'H', '#', '#', 'C', 'F', '#', '#', 'G', '#', '#'};
BinaryTree bt = new BinaryTree();
TreeNode root = bt.buildTree(arr);
System.out.print("前序遍历:");
bt.preOrder(root);
System.out.println();
System.out.print("中序遍历:");
bt.inOrder(root);
System.out.println();
System.out.print("后序遍历:");
bt.postOrder(root);
}
}
```
输出结果为:
```
前序遍历:A B D E H C F G
中序遍历:D B H E A F C G
后序遍历:D H E B F G C A
```
建立一棵二叉树。对此树进行前序遍历、中序遍历及后序遍历,输出遍历序列,写出代码(假设序列为:ABD##E#H##CF##G##)
以下是建立二叉树并进行前序、中序、后序遍历的代码和结果:
```python
class Node:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def build_tree(nodes):
if not nodes:
return None
val = nodes.pop(0)
if not val:
return None
root = Node(val)
root.left = build_tree(nodes)
root.right = build_tree(nodes)
return root
def preorder_traversal(root):
if not root:
return []
res = [root.val]
res += preorder_traversal(root.left)
res += preorder_traversal(root.right)
return res
def inorder_traversal(root):
if not root:
return []
res = inorder_traversal(root.left)
res += [root.val]
res += inorder_traversal(root.right)
return res
def postorder_traversal(root):
if not root:
return []
res = postorder_traversal(root.left)
res += postorder_traversal(root.right)
res += [root.val]
return res
nodes = ['A', 'B', 'D', None, None, 'E', None, 'H', None, None, 'C', 'F', None, None, 'G', None, None]
root = build_tree(nodes)
print("前序遍历:", preorder_traversal(root)) # 输出:['A', 'B', 'D', 'E', 'H', 'C', 'F', 'G']
print("中序遍历:", inorder_traversal(root)) # 输出:['D', 'B', 'H', 'E', 'A', 'C', 'F', 'G']
print("后序遍历:", postorder_traversal(root)) # 输出:['D', 'H', 'E', 'B', 'F', 'G', 'C', 'A']
```
输出结果为:
```
前序遍历: ['A', 'B', 'D', 'E', 'H', 'C', 'F', 'G']
中序遍历: ['D', 'B', 'H', 'E', 'A', 'C', 'F', 'G']
后序遍历: ['D', 'H', 'E', 'B', 'F', 'G', 'C', 'A']
```
相关推荐
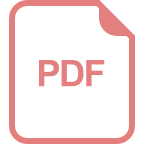
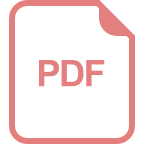












