opencv c++给视频加上椒盐噪声和高斯噪声
时间: 2023-12-31 13:05:28 浏览: 237
给视频加上椒盐噪声和高斯噪声可以分为以下几个步骤:
1. 读入视频文件
```c++
cv::VideoCapture cap("path/to/video/file");
```
2. 循环遍历视频的每一帧,并对每一帧添加噪声
```c++
while (cap.isOpened()) {
cv::Mat frame;
cap >> frame;
// 添加椒盐噪声
cv::Mat salt_pepper_noise = addSaltPepperNoise(frame, 0.01); // 噪声密度为0.01
// 添加高斯噪声
cv::Mat gaussian_noise = addGaussianNoise(frame, 0, 20); // 均值为0,标准差为20
// 显示加噪声后的图像
cv::imshow("Salt and Pepper Noise", salt_pepper_noise);
cv::imshow("Gaussian Noise", gaussian_noise);
// 等待键盘输入,按下ESC键退出循环
if (cv::waitKey(1) == 27) {
break;
}
}
```
3. 编写函数实现添加噪声的功能
添加椒盐噪声的函数:
```c++
cv::Mat addSaltPepperNoise(const cv::Mat& image, float density) {
cv::Mat result = image.clone();
int height = result.rows;
int width = result.cols;
int channels = result.channels();
int num_salt_pixels = static_cast<int>(density * height * width);
int num_pepper_pixels = static_cast<int>(density * height * width);
for (int i = 0; i < num_salt_pixels; i++) {
int row = std::rand() % height;
int col = std::rand() % width;
if (channels == 1) { // 灰度图像
result.at<uchar>(row, col) = 255;
} else if (channels == 3) { // 彩色图像
result.at<cv::Vec3b>(row, col)[0] = 255;
result.at<cv::Vec3b>(row, col)[1] = 255;
result.at<cv::Vec3b>(row, col)[2] = 255;
}
}
for (int i = 0; i < num_pepper_pixels; i++) {
int row = std::rand() % height;
int col = std::rand() % width;
if (channels == 1) { // 灰度图像
result.at<uchar>(row, col) = 0;
} else if (channels == 3) { // 彩色图像
result.at<cv::Vec3b>(row, col)[0] = 0;
result.at<cv::Vec3b>(row, col)[1] = 0;
result.at<cv::Vec3b>(row, col)[2] = 0;
}
}
return result;
}
```
添加高斯噪声的函数:
```c++
cv::Mat addGaussianNoise(const cv::Mat& image, double mean, double stddev) {
cv::Mat result = image.clone();
int height = result.rows;
int width = result.cols;
int channels = result.channels();
cv::Mat noise(height, width, CV_64FC(channels));
cv::randn(noise, mean, stddev);
cv::Mat noisy_image;
result.convertTo(noisy_image, CV_64FC(channels));
noisy_image += noise;
noisy_image.convertTo(result, image.type());
return result;
}
```
注意:在使用 `std::rand()` 生成随机数之前,需要调用 `std::srand()` ,并传入一个种子值。例如:
```c++
std::srand(static_cast<unsigned int>(std::time(nullptr)));
```
阅读全文
相关推荐
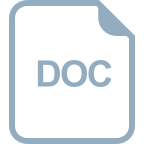
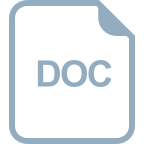
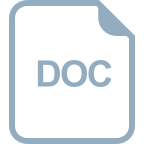















