opencv c++给视频添加椒盐噪声和高斯噪声
时间: 2023-09-04 08:15:40 浏览: 73
添加椒盐噪声可以使用 `cv::randu` 函数生成随机数,再根据一定的概率将像素点设置为黑色或白色,从而模拟椒盐噪声。
代码示例:
```c++
cv::Mat addSaltPepperNoise(const cv::Mat& image, float noise_ratio) {
cv::Mat result = image.clone();
cv::randu(result, 0, 255);
int noise_pixels = noise_ratio * image.cols * image.rows;
for (int i = 0; i < noise_pixels; i++) {
int x = std::rand() % image.cols;
int y = std::rand() % image.rows;
if (std::rand() % 2 == 0) {
result.at<uchar>(y, x) = 0;
} else {
result.at<uchar>(y, x) = 255;
}
}
return result;
}
```
其中,`image` 是输入的图像,`noise_ratio` 是添加噪声的比例,取值范围为 [0, 1]。
添加高斯噪声可以使用 `cv::randn` 函数生成随机数,再将随机数加到像素点上,从而模拟高斯噪声。
代码示例:
```c++
cv::Mat addGaussianNoise(const cv::Mat& image, float mean, float stddev) {
cv::Mat result = image.clone();
cv::randn(result, mean, stddev);
result = result.reshape(1, image.rows);
cv::Mat noise_image = image + result;
cv::normalize(noise_image, noise_image, 0, 255, cv::NORM_MINMAX, CV_8UC1);
return noise_image;
}
```
其中,`image` 是输入的图像,`mean` 和 `stddev` 分别是高斯分布的均值和标准差。
相关推荐
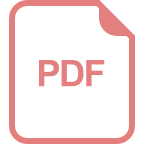
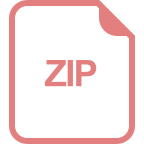














