c++语言实现稀疏矩阵类
时间: 2023-08-16 12:03:33 浏览: 65
好的,以下是一个简单的用C++实现稀疏矩阵类的示例代码:
```
#include <iostream>
#include <vector>
using namespace std;
class SparseMatrix {
private:
int rows, cols;
vector<vector<int>> data;
public:
SparseMatrix(int m, int n) {
rows = m;
cols = n;
}
void setValue(int row, int col, int value) {
if (row > rows || col > cols) {
cout << "Index out of range" << endl;
return;
}
if (data.empty()) {
vector<int> temp(cols, 0);
data.resize(rows, temp);
}
data[row - 1][col - 1] = value;
}
int getValue(int row, int col) {
if (row > rows || col > cols) {
cout << "Index out of range" << endl;
return -1;
}
if (data.empty() || data[row - 1][col - 1] == 0) {
return 0;
}
return data[row - 1][col - 1];
}
};
int main() {
SparseMatrix matrix(3, 3);
matrix.setValue(1, 1, 1);
matrix.setValue(1, 3, 2);
matrix.setValue(2, 2, 3);
matrix.setValue(3, 1, 4);
matrix.setValue(3, 3, 5);
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
cout << matrix.getValue(i, j) << " ";
}
cout << endl;
}
return 0;
}
```
在这个示例代码中,SparseMatrix类包含了三个私有成员:rows表示矩阵的行数,cols表示矩阵的列数,data则是一个二维vector,存储了矩阵中非零元素的值。SparseMatrix类提供了两个公有成员函数:setValue用于设置矩阵中某个元素的值,getValue用于获取矩阵中某个元素的值。其中,setValue函数会自动调整data的大小,以保证其能够存储所有非零元素的值。
在main函数中,我们创建了一个3x3的稀疏矩阵,然后设置了其中的五个元素,最后通过getValue函数来获取矩阵中各个元素的值,并输出结果。
相关推荐
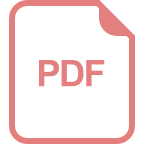
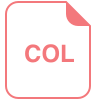

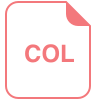
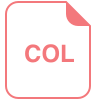










