迪杰斯特拉算法c语言
时间: 2023-08-24 12:22:02 浏览: 80
以下是一个简单的 C 语言实现迪杰斯特拉算法的代码示例:
```c
#include<stdio.h>
#define INF 9999
#define MAX 10
void dijkstra(int G[MAX][MAX], int n, int startnode);
int main() {
int G[MAX][MAX], i, j, n, u;
printf("Enter the number of vertices:");
scanf("%d", &n);
printf("\nEnter the adjacency matrix:\n");
for(i = 0; i < n; i++)
for(j = 0; j < n; j++)
scanf("%d", &G[i][j]);
printf("\nEnter the starting node:");
scanf("%d", &u);
dijkstra(G, n, u);
return 0;
}
void dijkstra(int G[MAX][MAX], int n, int startnode) {
int cost[MAX][MAX], distance[MAX], pred[MAX];
int visited[MAX], count, mindistance, nextnode, i, j;
// Creating cost matrix
for(i = 0; i < n; i++)
for(j = 0; j < n; j++)
if(G[i][j] == 0)
cost[i][j] = INF;
else
cost[i][j] = G[i][j];
// Initializing visited[], distance[], and pred[]
for(i = 0; i < n; i++) {
distance[i] = cost[startnode][i];
pred[i] = startnode;
visited[i] = 0;
}
distance[startnode] = 0;
visited[startnode] = 1;
count = 1;
while(count < n - 1) {
mindistance = INF;
// Nextnode gives the node at minimum distance
for(i = 0; i < n; i++)
if(distance[i] < mindistance && !visited[i]) {
mindistance = distance[i];
nextnode = i;
}
// Checking if a better path exists through nextnode
visited[nextnode] = 1;
for(i = 0; i < n; i++)
if(!visited[i])
if(mindistance + cost[nextnode][i] < distance[i]) {
distance[i] = mindistance + cost[nextnode][i];
pred[i] = nextnode;
}
count++;
}
// Printing the distance and path of each node
for(i = 0; i < n; i++)
if(i != startnode) {
printf("\nDistance of node %d = %d", i, distance[i]);
printf("\nPath = %d", i);
j = i;
do {
j = pred[j];
printf("<-%d", j);
} while(j != startnode);
}
}
```
注意:此代码仅为示例,实际应用中需要根据具体情况进行适当修改。
阅读全文
相关推荐
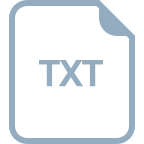






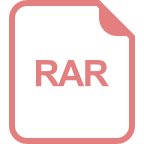




