用Python编写一个飞机游戏
时间: 2023-03-19 14:22:05 浏览: 160
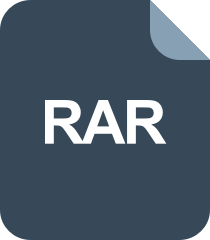
Python编写的打飞机游戏
好的,下面是一个简单的使用 Python 和 Pygame 库编写的飞机游戏示例。
首先,需要安装 Pygame 库。可以使用以下命令在终端或命令提示符下安装:
```
pip install pygame
```
然后,我们需要导入必要的库和模块:
```python
import pygame
import random
```
接下来,我们需要设置一些常量和变量:
```python
# 游戏窗口的大小
WIDTH = 500
HEIGHT = 600
# 颜色常量
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
# 初始化 Pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
# 设置游戏窗口标题
pygame.display.set_caption("飞机大战")
# 加载背景图片
background_image = pygame.image.load("background.jpg")
# 加载飞机图片
player_image = pygame.image.load("player.png")
player_width = player_image.get_width()
player_height = player_image.get_height()
# 加载敌机图片
enemy_image = pygame.image.load("enemy.png")
enemy_width = enemy_image.get_width()
enemy_height = enemy_image.get_height()
# 设置字体
font = pygame.font.SysFont(None, 30)
# 初始化计分
score = 0
```
然后,我们需要定义一些函数来处理游戏中的事件和动作:
```python
def draw_text(text, color, x, y):
"""在游戏窗口中绘制文本"""
text_surface = font.render(text, True, color)
screen.blit(text_surface, (x, y))
def draw_player(x, y):
"""在游戏窗口中绘制玩家飞机"""
screen.blit(player_image, (x, y))
def draw_enemy(x, y):
"""在游戏窗口中绘制敌机"""
screen.blit(enemy_image, (x, y))
def is_collision(player_x, player_y, enemy_x, enemy_y):
"""检测玩家飞机与敌机是否发生碰撞"""
distance = ((player_x - enemy_x) ** 2 + (player_y - enemy_y) ** 2) ** 0.5
if distance < (player_height + enemy_height) / 2:
return True
else:
return False
def game_over():
"""游戏结束"""
draw_text("游戏结束", RED, WIDTH // 2 - 50, HEIGHT // 2)
pygame.display.update()
pygame.time.delay(2000)
pygame.quit()
quit()
```
接下来,我们需要实现主游戏循环:
```python
# 初始化玩家飞机位置
player_x = WIDTH // 2 - player_width // 2
player_y = HEIGHT - player_height - 10
# 初始化敌机位置和速度
enemy_x = random.randint(0, WIDTH - enemy_width)
enemy_y = -enemy_height
enemy_speed = 5
# 设置游戏循环标志
running = True
# 开始游戏循环
while running:
# 处理游戏事件
阅读全文
相关推荐



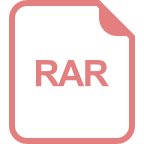






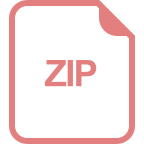
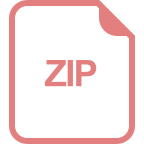
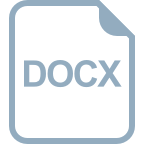
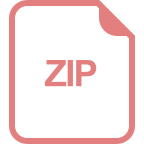
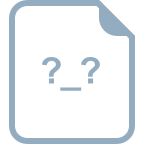
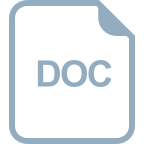