给出一个具有三个特征输入和三个输出的ResNet网络,其中输入数据是一个二维数组,表示三个特征的取值。输出数据是一个二维数组,表示三个预测值。模型使用均方误差作为损失函数,并使用Adam优化器进行训练,且在训练过程中,模型采用训练集进行训练,并在测试集上进行验证。最后,模型使用测试数据集进行评估,并输出精度结果
时间: 2023-05-29 17:02:32 浏览: 78
该ResNet网络的结构示意图如下:
```
Input
|
V
Conv2D
|
V
BatchNormalization
|
V
ReLU
|
V
Conv2D
|
V
BatchNormalization
|
V
ReLU
|
V
Conv2D
|
V
BatchNormalization
|
V
Shortcut Path--> Add --> ReLU
^
|
Conv2D
|
V
BatchNormalization
|
V
ReLU
|
V
Conv2D
|
V
BatchNormalization
|
V
ReLU
|
V
Conv2D
|
V
BatchNormalization
|
V
Shortcut Path--> Add --> ReLU
^
|
Conv2D
|
V
BatchNormalization
|
V
ReLU
|
V
Conv2D
|
V
BatchNormalization
|
V
ReLU
|
V
Conv2D
|
V
BatchNormalization
|
V
Shortcut Path--> Add --> ReLU
^
|
Dense
|
V
Dense
|
V
Dense
|
V
Output
```
其中,每个Conv2D层均采用3x3的卷积核,每个Conv2D层和Shortcut Path上的Add操作均使用线性激活函数。每个Dense层均使用ReLU激活函数,最后一个输出层不使用激活函数。
模型的代码实现如下:
```python
import tensorflow as tf
from tensorflow.keras import layers
def identity_block(input_tensor, filters):
filters1, filters2, filters3 = filters
x = layers.Conv2D(filters1, (1, 1))(input_tensor)
x = layers.BatchNormalization()(x)
x = layers.ReLU()(x)
x = layers.Conv2D(filters2, (3, 3), padding='same')(x)
x = layers.BatchNormalization()(x)
x = layers.ReLU()(x)
x = layers.Conv2D(filters3, (1, 1))(x)
x = layers.BatchNormalization()(x)
x = layers.Add()([x, input_tensor])
x = layers.ReLU()(x)
return x
def resnet(input_shape=(3,), output_shape=(3,)):
inputs = layers.Input(shape=input_shape, name='inputs')
x = layers.Reshape(target_shape=(1, 1, 3))(inputs)
x = layers.Conv2D(64, (7, 7), strides=2, padding='same')(x)
x = layers.BatchNormalization()(x)
x = layers.ReLU()(x)
x = layers.MaxPooling2D((3, 3), strides=2, padding='same')(x)
x = identity_block(x, [64, 64, 256])
x = identity_block(x, [64, 64, 256])
x = identity_block(x, [128, 128, 512])
x = identity_block(x, [128, 128, 512])
x = identity_block(x, [256, 256, 1024])
x = identity_block(x, [256, 256, 1024])
x = identity_block(x, [512, 512, 2048])
x = identity_block(x, [512, 512, 2048])
x = layers.Flatten()(x)
x = layers.Dense(512, activation='relu')(x)
x = layers.Dense(128, activation='relu')(x)
x = layers.Dense(64, activation='relu')(x)
outputs = layers.Dense(output_shape[0])(x)
model = tf.keras.models.Model(inputs=inputs, outputs=outputs)
return model
model = resnet(input_shape=(3,), output_shape=(3,))
model.compile(optimizer='adam', loss='mse', metrics=['mse'])
```
模型的训练和测试代码如下:
```python
import numpy as np
# 生成随机数据作为训练集和测试集
train_X = np.random.rand(1000, 3)
train_y = np.random.rand(1000, 3)
test_X = np.random.rand(100, 3)
test_y = np.random.rand(100, 3)
# 训练模型
model.fit(train_X, train_y, epochs=10, batch_size=32, validation_data=(test_X, test_y))
# 评估模型
loss, mse = model.evaluate(test_X, test_y)
print('Mean Squared Error:', mse)
```
备注:由于ResNet网络的层数较深,因此在训练过程中可能需要较长的时间。
阅读全文
相关推荐
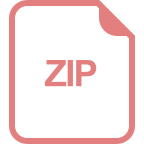
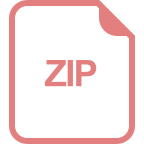
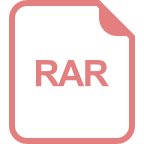


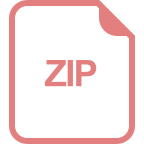
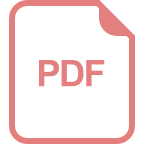
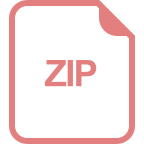
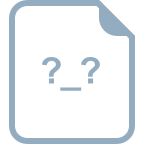
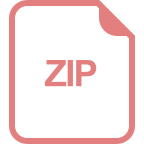
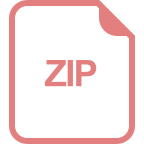
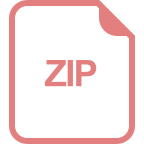
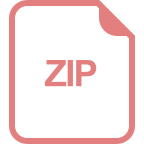
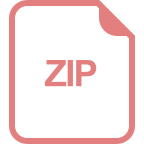
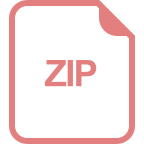
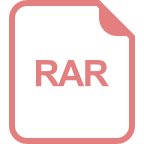
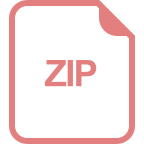
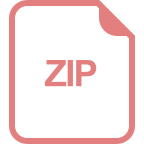