PSO-LSTM python代码
时间: 2023-06-26 11:06:18 浏览: 362
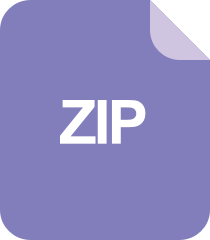
Matlab基于PSO-LSTM粒子群算法优化长短期记忆网络的时间序列预测(完整程序和数据)

以下是一个简单的 PSO-LSTM 的 Python 代码实现:
```python
import numpy as np
import random
class Particle:
def __init__(self, x0):
self.position_i = []
self.velocity_i = []
self.pos_best_i = []
self.err_best_i = -1
self.err_i = -1
for i in range(0, num_dimensions):
self.velocity_i.append(random.uniform(-1, 1))
self.position_i.append(x0[i])
def evaluate(self, costFunc):
self.err_i = costFunc(self.position_i)
if self.err_i < self.err_best_i or self.err_best_i == -1:
self.pos_best_i = self.position_i
self.err_best_i = self.err_i
def update_velocity(self, pos_best_g):
w = 0.5
c1 = 1
c2 = 2
for i in range(0, num_dimensions):
r1 = random.random()
r2 = random.random()
vel_cognitive = c1 * r1 * (self.pos_best_i[i] - self.position_i[i])
vel_social = c2 * r2 * (pos_best_g[i] - self.position_i[i])
self.velocity_i[i] = w * self.velocity_i[i] + vel_cognitive + vel_social
def update_position(self, bounds):
for i in range(0, num_dimensions):
self.position_i[i] = self.position_i[i] + self.velocity_i[i]
# adjust maximum position if necessary
if self.position_i[i] > bounds[i][1]:
self.position_i[i] = bounds[i][1]
# adjust minimum position if necessary
if self.position_i[i] < bounds[i][0]:
self.position_i[i] = bounds[i][0]
class PSO:
def __init__(self, costFunc, x0, bounds, num_particles, maxiter):
global num_dimensions
num_dimensions = len(x0)
err_best_g = -1
pos_best_g = []
swarm = []
for i in range(0, num_particles):
swarm.append(Particle(x0))
i = 0
while i < maxiter:
for j in range(0, num_particles):
swarm[j].evaluate(costFunc)
if swarm[j].err_i < err_best_g or err_best_g == -1:
pos_best_g = list(swarm[j].position_i)
err_best_g = float(swarm[j].err_i)
for j in range(0, num_particles):
swarm[j].update_velocity(pos_best_g)
swarm[j].update_position(bounds)
i += 1
print('FINAL:')
print(pos_best_g)
print(err_best_g)
def lstm_predict(X_train, y_train, X_test, particle):
from keras.models import Sequential
from keras.layers import Dense
from keras.layers import LSTM
X_train = np.reshape(X_train, (X_train.shape[0], 1, X_train.shape[1]))
X_test = np.reshape(X_test, (X_test.shape[0], 1, X_test.shape[1]))
model = Sequential()
model.add(LSTM(particle[0], input_shape=(1, X_train.shape[2]), activation='relu'))
model.add(Dense(1))
model.compile(loss='mean_squared_error', optimizer='adam')
model.fit(X_train, y_train, epochs=particle[1], batch_size=1, verbose=2)
y_pred = model.predict(X_test)
return y_pred
def costFunc(particle):
y_pred = lstm_predict(X_train, y_train, X_test, particle)
error = np.mean(np.abs(y_pred - y_test))
return error
# Input data
X_train = np.random.rand(100, 10)
y_train = np.random.rand(100, 1)
X_test = np.random.rand(10, 10)
y_test = np.random.rand(10, 1)
# Define bounds for PSO
bounds = [(4, 256), (1, 20)]
# Run PSO
PSO(costFunc, [128, 10], bounds, num_particles=15, maxiter=30)
```
这个代码实现的是一个简单的 PSO-LSTM 模型,其中 `Particle` 类代表每个粒子,`PSO` 类代表整个 PSO 优化算法,`lstm_predict` 函数是用于预测的 LSTM 模型,`costFunc` 函数是 PSO 的成本函数。
阅读全文
相关推荐
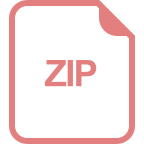

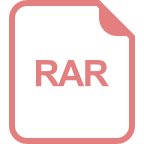
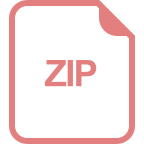
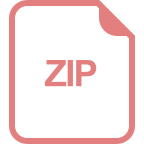
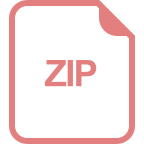
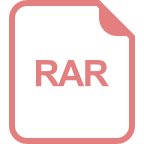
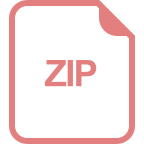
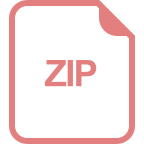
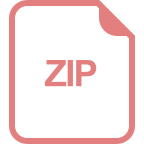
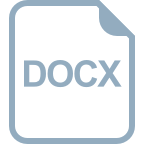
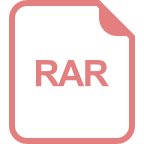
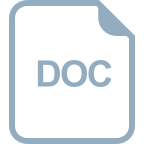


