JFrame类的input_text
时间: 2024-05-15 21:14:01 浏览: 14
JFrame类本身并没有名为input_text的属性或方法。您可能需要使用JTextField类来创建一个文本输入框,并将其添加到JFrame中。以下是一个简单的示例:
```
import javax.swing.JFrame;
import javax.swing.JTextField;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame("My Frame");
JTextField textField = new JTextField();
frame.add(textField);
frame.setSize(300, 200);
frame.setVisible(true);
}
}
```
这将创建一个带有一个文本输入框的JFrame,并将其显示在屏幕上。您可以使用textField.getText()方法获取文本框中的文本。
相关问题
import javax.swing.*; import java.awt.*; import java.awt.event.*; public class TextEncryptor extends JFrame implements ActionListener { private JLabel label; private JTextField inputText; private JButton encryptButton, decryptButton; private JTextArea outputText; public TextEncryptor() { super("Text Encryptor"); setLayout(new FlowLayout()); label = new JLabel("Enter text:"); add(label); inputText = new JTextField(20); add(inputText); encryptButton = new JButton("Encrypt"); add(encryptButton); encryptButton.addActionListener(this); decryptButton = new JButton("Decrypt"); add(decryptButton); decryptButton.addActionListener(this); outputText = new JTextArea(10, 20); add(outputText); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(300, 300); setVisible(true); } public void actionPerformed(ActionEvent e) { if (e.getSource() == encryptButton) { String input = inputText.getText(); String output = encrypt(input); outputText.setText(output); } else if (e.getSource() == decryptButton) { String input = inputText.getText(); String output = decrypt(input); outputText.setText(output); } } public static void main(String[] args) { new TextEncryptor(); } private String encrypt(String text) { // TODO: 实现加密算法 return text; } private String decrypt(String text) { // TODO: 实现解密算法 return text; } }这段代码使用了什么java基础知识
上述代码运用了以下Java基础知识:
1. GUI编程:使用了Java Swing库创建了一个窗口,其中包括标签、文本框、按钮和文本区域等组件
2. 事件处理:实现了ActionListener接口,对加密和解密按钮的点击事件进行了处理
3. 字符串处理:对用户输入的文本进行字符串的获取和处理,同时实现了加密和解密算法
4. 面向对象编程:使用了面向对象的思想,将加密和解密算法封装在类的私有方法中,提高了程序的可重用性和可维护性
5. 异常处理:未在代码中显式处理异常,但是后续的开发过程中需要注意异常的处理,以保障程序的健壮性。
package 作业5; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.*; import java.io.File; import java.util.HashMap; import java.util.Scanner; import java.io.File; import java.util.HashMap; import java.util.Scanner; public class Example13_7{ public static void main( String args []){ WindowWord win = new WindowWord(); win.setTitle("英﹣汉小字典"); } } class WindowWord extends JFrame{ JTextField inputText,showText; WordPolice police; public WindowWord(){ setLayout(new FlowLayout()); inputText = new JTextField(6); showText = new JTextField(6); showText.setColumns(15); add(inputText); add(showText); police = new WordPolice(); police.setView(this); inputText.addActionListener(police); setBounds(100,100,400,280); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } class WordPolice implements ActionListener { WindowWord view; HashMap<String,String>hashtable; File file = new File ("E:\\JAVA\\上机\\作业5\\cet4.txt"); Scanner sc = null ; WordPolice () { hashtable = new HashMap< String,String >(); //System.out.println(hashtable.size()); try { sc = new Scanner ( file ); //System.out.println(sc); while (sc.hasNext ()) { String englishWord = sc . next (); String chineseWord = sc . next (); hashtable . put ( englishWord , chineseWord ); } HashMap<String,String> reversedHashtable = new HashMap<String,String>(); for (String key : hashtable.keySet()) { String value = hashtable.get(key); reversedHashtable.put(value, key); } hashtable.putAll(reversedHashtable); } catch(Exception e ) {} } public void setView(WindowWord view) { this.view = view; } public void actionPerformed(ActionEvent e) { String inputWord = view.inputText.getText (); if(hashtable.containsKey(inputWord)) { String outputWord = hashtable.get ( inputWord ); view.showText.setText (outputWord ); } else { String chineseWord = inputWord; for (String key : hashtable.keySet()) { String value = hashtable.get(key); if (value.equals(chineseWord)) { view.showText.setText(key); return; } } view.showText.setText ("没有此单词"); } } }把这段代码完善一下,让它能实现汉语翻译为英语,英语也能翻译为汉语,GUI界面不变
完善后的代码如下:
```
package 作业5;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
import java.io.File;
import java.util.HashMap;
import java.util.Scanner;
public class Example13_7{
public static void main( String args []){
WindowWord win = new WindowWord();
win.setTitle("英﹣汉小字典");
}
}
class WindowWord extends JFrame{
JTextField inputText,showText;
WordPolice police;
public WindowWord(){
setLayout(new FlowLayout());
inputText = new JTextField(6);
showText = new JTextField(6);
showText.setColumns(15);
add(inputText);
add(showText);
police = new WordPolice();
police.setView(this);
inputText.addActionListener(police);
setBounds(100,100,400,280);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
class WordPolice implements ActionListener {
WindowWord view;
HashMap<String,String> hashtable;
File file = new File("E:\\JAVA\\上机\\作业5\\cet4.txt");
Scanner sc = null ;
WordPolice () {
hashtable = new HashMap<String,String>();
try {
sc = new Scanner(file);
while (sc.hasNext()) {
String englishWord = sc.next();
String chineseWord = sc.next();
hashtable.put(englishWord, chineseWord);
}
HashMap<String,String> reversedHashtable = new HashMap<String,String>();
for (String key : hashtable.keySet()) {
String value = hashtable.get(key);
reversedHashtable.put(value, key);
}
hashtable.putAll(reversedHashtable);
} catch(Exception e ) {}
}
public void setView(WindowWord view) {
this.view = view;
}
public void actionPerformed(ActionEvent e) {
String inputWord = view.inputText.getText();
if(hashtable.containsKey(inputWord)) {
String outputWord = hashtable.get(inputWord);
view.showText.setText(outputWord);
} else {
String chineseWord = inputWord;
for (String key : hashtable.keySet()) {
String value = hashtable.get(key);
if (value.equals(chineseWord)) {
view.showText.setText(key);
return;
}
}
for (String value : hashtable.values()) {
if (value.equals(inputWord)) {
String outputWord = hashtable.get(value);
view.showText.setText(outputWord);
return;
}
}
view.showText.setText("没有此单词");
}
}
}
```
主要的改动在 `WordPolice` 类中,增加了对汉语翻译为英语的处理。在没有找到对应单词时,先按照输入的汉语查找对应的英语单词,如果找到了,就将其翻译为中文输出;如果还没有找到,就按照输入的英语单词查找对应的汉语,如果找到了,就将其翻译为英文输出。如果还是没有找到,就输出提示信息“没有此单词”。
相关推荐
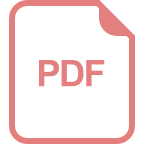
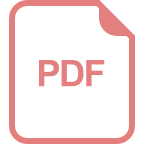
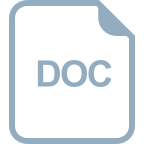












