假设一个算术表达式中包含圆括号,方括号和花括号三种类型的括号,用C语言编写一个程序,用来判别表达式中的括号是否正确匹配
时间: 2024-05-10 10:21:38 浏览: 265
思路:
1. 创建一个栈来存储左括号,遍历表达式中的每个字符,如果是左括号则入栈,如果是右括号则判断是否和栈顶元素匹配,如果匹配则弹出栈顶元素,否则返回括号不匹配。
2. 如果遍历完整个表达式后栈不为空,则返回括号不匹配。
代码实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX_SIZE 100
int top = -1; // 栈顶指针
char stack[MAX_SIZE]; // 栈
void push(char c)
{
if (top == MAX_SIZE - 1) {
printf("Stack Overflow\n");
return;
}
stack[++top] = c;
}
char pop()
{
if (top == -1) {
printf("Stack Underflow\n");
return '\0';
}
return stack[top--];
}
int isMatchingPair(char left, char right)
{
if (left == '(' && right == ')')
return 1;
else if (left == '[' && right == ']')
return 1;
else if (left == '{' && right == '}')
return 1;
else
return 0;
}
int areParenthesesBalanced(char* exp)
{
int len = strlen(exp);
for (int i = 0; i < len; i++) {
if (exp[i] == '(' || exp[i] == '[' || exp[i] == '{')
push(exp[i]);
else if (exp[i] == ')' || exp[i] == ']' || exp[i] == '}') {
if (top == -1)
return 0;
else if (!isMatchingPair(pop(), exp[i]))
return 0;
}
}
if (top == -1)
return 1;
else
return 0;
}
int main()
{
char exp[MAX_SIZE];
printf("Enter an expression: ");
scanf("%s", exp);
if (areParenthesesBalanced(exp))
printf("Parentheses are balanced\n");
else
printf("Parentheses are not balanced\n");
return 0;
}
```
测试:
输入:`{[(a+b)*c]-(d+e)/f}`
输出:`Parentheses are balanced`
输入:`{[(a+b)*c-(d+e)/f}`
输出:`Parentheses are not balanced`
阅读全文
相关推荐
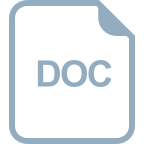
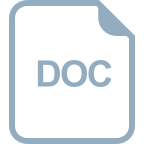
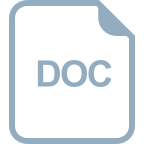
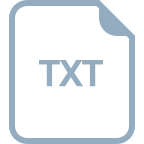
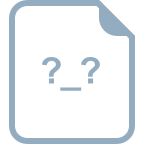
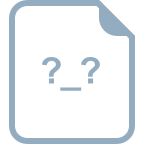
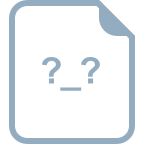
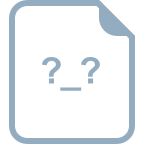
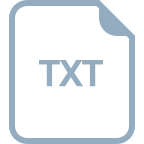
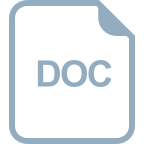
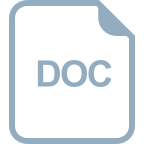
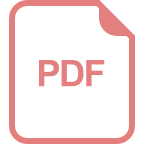