蚁群算法旅行商问题Python
时间: 2023-08-14 10:14:28 浏览: 41
以下是使用Python实现蚁群算法解决旅行商问题的代码:
```python
import random
class Ant:
def __init__(self, n, alpha, beta, pheromone, distance):
self.n = n
self.alpha = alpha
self.beta = beta
self.pheromone = pheromone
self.distance = distance
self.visited = [False] * n
self.path = []
self.total_distance = 0
def select_next_city(self, current_city):
unvisited_cities = [i for i in range(self.n) if not self.visited[i]]
if not unvisited_cities:
return None
probabilities = [self.pheromone[current_city][i] ** self.alpha *
(1.0 / self.distance[current_city][i]) ** self.beta for i in unvisited_cities]
total = sum(probabilities)
r = random.uniform(0, total)
prob_sum = 0
for i, city in enumerate(unvisited_cities):
prob_sum += probabilities[i]
if prob_sum >= r:
return city
def visit_city(self, city):
self.visited[city] = True
self.path.append(city)
if len(self.path) == 1:
self.total_distance = 0
else:
prev = self.path[-2]
self.total_distance += self.distance[prev][city]
def __str__(self):
return f'{self.path} ({self.total_distance})'
class AntColony:
def __init__(self, distances, n_ants, n_best, n_iterations, decay, alpha=1, beta=1):
self.distances = distances
self.pheromone = [[1.0 / (distances[i][j]) for j in range(n)] for i in range(n)]
self.all_ants = []
for i in range(n_ants):
self.all_ants.append(Ant(n, alpha, beta, self.pheromone, distances))
self.n_best = n_best
self.n_iterations = n_iterations
self.decay = decay
self.best_ant = None
self.best_distance = float('inf')
self.average_distance = 0
def run(self):
for iteration in range(self.n_iterations):
for ant in self.all_ants:
start = random.randint(0, self.n - 1)
ant.visited = [False] * self.n
ant.path = []
ant.total_distance = 0
ant.visit_city(start)
for i in range(self.n - 1):
current_city = ant.path[-1]
next_city = ant.select_next_city(current_city)
if next_city is None:
break
ant.visit_city(next_city)
if ant.total_distance < self.best_distance:
self.best_distance = ant.total_distance
self.best_ant = ant
for i in range(1, len(ant.path)):
prev = ant.path[i - 1]
current = ant.path[i]
self.pheromone[prev][current] += 1.0 / ant.total_distance
self.pheromone[current][prev] = self.pheromone[prev][current]
self.average_distance += ant.total_distance
self.average_distance /= len(self.all_ants)
for i, row in enumerate(self.pheromone):
for j, value in enumerate(row):
self.pheromone[i][j] *= self.decay
self.pheromone[i][j] = max(self.pheromone[i][j], 0.0001)
return self.best_ant
```
这段代码实现了Ant和AntColony类,其中Ant类表示一只蚂蚁,AntColony类管理整个蚁群算法的运行。在Ant类中,我们实现了选择下一个城市和访问城市的方法。在AntColony类中,我们使用了蚁群算法的基本步骤:初始化信息素、移动蚂蚁并更新信息素、更新最优解和信息素浓度、重复执行多次以找到最优解。
使用这段代码解决旅行商问题的步骤如下:
1. 创建一个n×n的距离矩阵,其中第i行第j列表示从城市i到城市j的距离。
2. 创建一个AntColony对象,并传入距离矩阵、蚂蚁数量、最优解数量、迭代次数、信息素挥发率等参数。
3. 调用AntColony的run()方法,得到最优解Ant对象。
4. 从Ant对象中获取路径和距离,即可得到最优解。
以下是一个简单的示例:
```python
n = 5
distances = [[0, 2, 3, 4, 5],
[2, 0, 4, 5, 6],
[3, 4, 0, 6, 7],
[4, 5, 6, 0, 8],
[5, 6, 7, 8, 0]]
colony = AntColony(distances, n_ants=10, n_best=2, n_iterations=10, decay=0.1)
best_ant = colony.run()
print(best_ant)
```
这个示例中,我们创建了一个5个城市的旅行商问题,距离矩阵为distances。我们创建了一个AntColony对象,设置蚂蚁数量为10,最优解数量为2,迭代次数为10,信息素挥发率为0.1。最后,我们调用run()方法得到最优解,并打印出来。
相关推荐
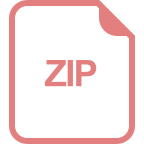
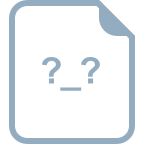














