asyncio python 例子
时间: 2023-12-09 20:37:20 浏览: 80
以下是一个使用asyncio和Kubernetes Python客户端API的例子,用于列出所有的Pods:
```python
import asyncio
from kubernetes_asyncio import client, config
from kubernetes_asyncio.client.api_client import ApiClient
async def main():
# 配置
config.load_kube_config()
# 创建API客户端
api_client = ApiClient()
# 创建API实例
v1 = client.CoreV1Api(api_client)
# 获取所有的Pods
ret = await v1.list_pod_for_all_namespaces()
# 打印每个Pod的名称
for i in ret.items:
print(i.metadata.name)
asyncio.run(main())
```
相关问题
asyncio python
您好!对于您的问题关于 asyncio 在 Python 中的使用,我很乐意为您提供帮助。
asyncio 是 Python 中的一个用于异步编程的库。它提供了一种编写并发代码的方式,可以通过协程(coroutines)和事件循环(event loop)来实现非阻塞的异步操作。
使用 asyncio,您可以将耗时的操作(如网络请求或文件读写)放在协程中,然后通过事件循环来调度执行这些协程。这样可以避免阻塞主线程,提高程序的性能和响应性。
下面是一个简单的示例代码,展示了如何使用 asyncio 进行异步操作:
```python
import asyncio
async def hello():
print("Hello")
await asyncio.sleep(1)
print("World")
async def main():
await asyncio.gather(hello(), hello(), hello())
if __name__ == "__main__":
asyncio.run(main())
```
在上面的代码中,我们定义了一个名为 `hello` 的协程函数,它会打印 "Hello",然后等待 1 秒后再打印 "World"。然后我们定义了一个名为 `main` 的协程函数,它使用 `asyncio.gather` 来同时运行多个 `hello` 协程。
最后,在主程序中,我们使用 `asyncio.run` 来运行 `main` 协程。
请注意,为了在 Python 3.7 之前的版本中使用 asyncio,您需要引入 `asyncio` 库,并使用 `asyncio.get_event_loop().run_until_complete(main())` 来运行主协程。
希望这个例子能帮助到您!如果您有任何进一步的问题,请随时提问。
python asyncio subprocess用法例子
好的,以下是一段python asyncio subprocess的用法例子:
```python
import asyncio
async def run_command(cmd):
proc = await asyncio.create_subprocess_shell(cmd, stdout=asyncio.subprocess.PIPE, stderr=asyncio.subprocess.PIPE)
stdout, stderr = await proc.communicate()
return (stdout.decode(), stderr.decode())
async def main():
cmd = "ls -al"
result = await run_command(cmd)
print(result[0])
asyncio.run(main())
```
这段代码使用了Python的asyncio库,并创建了一个异步函数`run_command`来执行一个shell命令。在`main`函数中,使用`asyncio.run`运行了`run_command`函数,并且打印了返回的标准输出。这是一个简单的例子,你可以根据自己的需要,修改命令和返回值,并添加更多的异步函数来执行更复杂的任务。
阅读全文
相关推荐
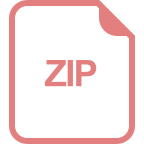
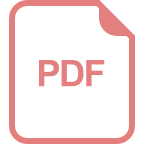
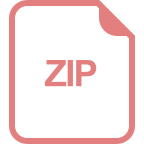













