rtime = time.strftime("%Y-%m-%d %H:%M:%S", time_local)
时间: 2023-09-09 13:13:27 浏览: 116
这行代码是使用 Python 中的 `time` 模块对时间进行格式化。`time_local` 是一个 `time.struct_time` 类型的对象,表示本地时间,`"%Y-%m-%d %H:%M:%S"` 是一个时间格式化的字符串。
具体来说,`%Y` 表示年份,`%m` 表示月份,`%d` 表示日期,`%H` 表示小时,`%M` 表示分钟,`%S` 表示秒数。这些占位符会被替换成对应的时间值。例如,`"%Y-%m-%d %H:%M:%S"` 表示将时间格式化为类似于 `2022-01-01 12:30:45` 的字符串。
`time.strftime` 方法接受两个参数,第一个参数是时间格式化的字符串,第二个参数是一个 `time.struct_time` 类型的对象。该方法将 `time.struct_time` 类型的对象转换成指定格式的时间字符串,并返回该字符串。
因此,这行代码的作用是将 `time_local` 表示的本地时间对象转换成指定格式的时间字符串,并将结果存储到 `rtime` 变量中。
相关问题
<%@ page language="java" import="java.util.*, java.sql.*" pageEncoding="utf-8"%> <%@page import="com.util.SQLConn"%> <%@page import="org.apache.commons.lang3.*" %> <%@page import="com.util.LOGS" %> <html> <body> <%-- 获取前端数据 --%> <% String username = request.getParameter("username"); String usernum = request.getParameter("usernum"); String name=request.getParameter("ytime"); String mon=request.getParameter("mtime"); String num=request.getParameter("rtime"); String people = request.getParameter("people"); %> <%-- 定义SQLConn类和INSERT_SQL常量 --%> <% final String INSERT_SQL = "INSERT INTO YuYue(UserID, Time, People) VALUES (?, ?, ?)"; %> <%-- 执行数据库操作 --%> <% int count = 0; try {Connection conn = SQLConn.openDB(); PreparedStatement pstmt = conn.prepareStatement(INSERT_SQL); { pstmt.setString(1, usernum); pstmt.setString(2, name+mon+num); pstmt.setString(3, people); count = pstmt.executeUpdate(); }} catch (SQLException e) { e.printStackTrace(); } %> <%-- 根据执行结果进行相应的操作 --%> <c:choose> <c:when test="${count > 0}"> <%-- 成功跳转到ZCS.jsp --%> <c:redirect url="ZCS.jsp"/> </c:when> <c:otherwise> <%-- 失败跳转到ZCB.jsp --%> <c:redirect url="../ZCB.jsp"/> </c:otherwise> </c:choose> </body> </html>优化代码
以下是对代码进行优化的建议:
1. 在第二行中,导入语句中的星号通配符应该避免使用,应该明确导入需要使用的类或接口。
2. 在第十行中,可以将 INSERT_SQL 常量定义在 SQLConn 类中并进行调用,以提高代码的可维护性和复用性。
3. 在第十二行中,PreparedStatement 对象应该使用 try-with-resources 语句,以自动释放资源并避免资源泄露问题。
4. 在第十六至二十二行中,可以使用 EL 表达式代替 JSTL 标签,以简化代码并提高可读性。
基于上述建议,优化后的代码如下:
```
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ page import="java.util.*, java.sql.*"%>
<%@ page import="com.util.SQLConn"%>
<%@ page import="com.util.LOGS"%>
<%@ page import="org.apache.commons.lang3.*"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert Data</title>
</head>
<body>
<%-- 获取前端数据 --%>
<% String username = request.getParameter("username");
String usernum = request.getParameter("usernum");
String name = request.getParameter("ytime");
String mon = request.getParameter("mtime");
String num = request.getParameter("rtime");
String people = request.getParameter("people"); %>
<%-- 执行数据库操作 --%>
<% int count = 0;
try (Connection conn = SQLConn.openDB();
PreparedStatement pstmt = conn.prepareStatement(SQLConn.INSERT_SQL)) {
pstmt.setString(1, usernum);
pstmt.setString(2, name + mon + num);
pstmt.setString(3, people);
count = pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} %>
<%-- 根据执行结果进行相应的操作 --%>
<c:if test="${count > 0}">
<%-- 成功跳转到 ZCS.jsp --%>
<jsp:forward page="ZCS.jsp"></jsp:forward>
</c:if>
<c:if test="${count <= 0}">
<%-- 失败跳转到 ZCB.jsp --%>
<jsp:forward page="../ZCB.jsp"></jsp:forward>
</c:if>
</body>
</html>
```
优化后的代码更加简洁、易读、易维护,并且避免了可能存在的一些问题。
将下面c语言代码改为python代码 #include<stdio.h> #include<stdlib.h> struct PROCESS { char PROCESS_NAME[10]; int atime; int rtime; int ttime; int X; float Response_ratio; }; int main() { struct PROCESS p[3]; for (int i=0;i<3;i++) { scanf("%s %d %d",&p[i].PROCESS_NAME,&p[i].atime,&p[i].rtime); p[i].X=i+1; } struct PROCESS temp; int nowtime=p[0].atime; int sign=0; int flag=0; int m=0; int n=0; for(int i=1;i<3;i++) { if(p[i].atime==nowtime) { if(p[i].rtime<p[sign].rtime) { sign=i; nowtime=p[i].atime; temp=p[i]; p[i]=p[0]; p[0]=temp; } } else if(p[i].atime<nowtime) { sign=i; nowtime=p[i].atime; temp=p[i]; p[i]=p[0]; p[0]=temp; } } while (1) { if(p[m].atime>=n) { p[m].ttime=p[m].rtime; n=p[m].atime+p[m].rtime; } else { p[m].ttime=p[m].rtime+n-p[m].atime; n+=p[m].rtime; } for(int i=1;i<3;i++) { p[i].Response_ratio=(float)(n-p[i].atime)/p[i].rtime; } m++; if(m==3) { break; } if(flag==0) { if(p[1].Response_ratio>=p[2].Response_ratio) { if(p[1].Response_ratio==p[2].Response_ratio) { if(p[1].X>p[2].X) { temp=p[1]; p[1]=p[2]; p[2]=temp; } } } else { temp=p[1]; p[1]=p[2]; p[2]=temp; } flag=1; } } for(int i=0;i<2;i++) { for(int j=1;j<3;j++) { if(p[i].X>p[j].X) { temp=p[i]; p[i]=p[j]; p[j]=temp; } } } for(int i=0;i<3;i++) { printf("%d ",p[i].ttime); } }
以下是将C语言代码转换为Python代码后的结果:
```python
class Process:
def __init__(self):
self.PROCESS_NAME = ""
self.atime = 0
self.rtime = 0
self.ttime = 0
self.X = 0
self.Response_ratio = 0.0
p = [Process() for i in range(3)]
for i in range(3):
p[i].PROCESS_NAME, p[i].atime, p[i].rtime = input().split()
p[i].atime = int(p[i].atime)
p[i].rtime = int(p[i].rtime)
p[i].X = i + 1
temp = Process()
nowtime = p[0].atime
sign = 0
flag = 0
m = 0
n = 0
for i in range(1, 3):
if p[i].atime == nowtime:
if p[i].rtime < p[sign].rtime:
sign = i
nowtime = p[i].atime
temp = p[i]
p[i] = p[0]
p[0] = temp
elif p[i].atime < nowtime:
sign = i
nowtime = p[i].atime
temp = p[i]
p[i] = p[0]
p[0] = temp
while True:
if p[m].atime >= n:
p[m].ttime = p[m].rtime
n = p[m].atime + p[m].rtime
else:
p[m].ttime = p[m].rtime + n - p[m].atime
n += p[m].rtime
for i in range(1, 3):
p[i].Response_ratio = (n - p[i].atime) / p[i].rtime
m += 1
if m == 3:
break
if flag == 0:
if p[1].Response_ratio >= p[2].Response_ratio:
if p[1].Response_ratio == p[2].Response_ratio:
if p[1].X > p[2].X:
temp = p[1]
p[1] = p[2]
p[2] = temp
else:
temp = p[1]
p[1] = p[2]
p[2] = temp
flag = 1
for i in range(2):
for j in range(1, 3):
if p[i].X > p[j].X:
temp = p[i]
p[i] = p[j]
p[j] = temp
for i in range(3):
print(p[i].ttime, end=' ')
```
阅读全文
相关推荐
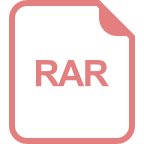
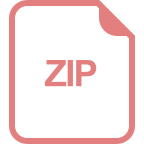














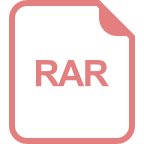