new Thread(() -> { for (int i = 0;i < maxStep;i++) { File file = new File(getFilesDir().toString() + File.separatorChar + fileName[i]); //上传数据 PostProcedure postProcedure = new PostProcedure(); JSONObject jsonObject = new JSONObject(); try { jsonObject.put("menuId", data); jsonObject.put("content", arrayList.get(i).getDetail()); jsonObject.put("file", file); } catch (JSONException e) { throw new RuntimeException(e); } Map map = null; try { map = postProcedure.postRequestProcedure("/menu/step/save", jsonObject); } catch (JSONException e) { throw new RuntimeException(e); } Map finalMap = map; runOnUiThread(() -> { if (finalMap == null) { ToastUtil.show(this, "网络异常"); } else { String message = (String) finalMap.get("message"); ToastUtil.show(this, message.equals("ok") ? "步骤上传成功!" : message); } }); } }).start();
时间: 2024-02-10 11:06:36 浏览: 28
这段代码是在Android平台中使用多线程的方式上传文件和数据到服务器。代码中使用了一个线程,循环上传多个文件和数据。具体步骤如下:
1. 创建一个新的线程,使用lambda表达式来实现Runnable接口的run()方法。
2. 在循环中,获取当前要上传的文件,创建一个PostProcedure对象,用于发送POST请求到服务器。
3. 构建一个JSONObject对象,用于封装要上传的数据。其中包括menuId、content和file三个字段。其中file字段是一个文件对象,用来上传文件数据。
4. 调用PostProcedure的postRequestProcedure()方法,将构建好的JSONObject对象作为参数传入,发送POST请求到服务器。
5. 在请求返回后,根据返回的结果显示相应的提示信息。如果上传成功,则弹出“步骤上传成功”提示框;否则,弹出“网络异常”或者具体的错误信息。
需要注意的是,由于Android平台中不允许在主线程中进行网络请求,因此需要在新的线程中发送请求。同时,由于上传文件和数据较为耗时,因此需要使用多线程的方式来实现异步上传,以避免阻塞主线程。
相关问题
public static void main(String[] args) throws IOException { Workbook workbook = WorkbookFactory.create(new File("lib/1.xlsx")); Sheet sheet = workbook.getSheetAt(0); int rowCount = sheet.getLastRowNum() + 1; int colCount = sheet.getRow(0).getLastCellNum(); int cellWidth = 100 / colCount; int cellHeight = 100 / rowCount; StringBuilder html = new StringBuilder(); html.append("<table>"); for (int i = 0; i < rowCount; i++) { html.append("<tr>"); for (int j = 0; j < colCount; j++) { Cell cell = sheet.getRow(i).getCell(j); String value = cell.getStringCellValue(); html.append("<td style="width: " + cellWidth + "%; height: " + cellHeight + "%;">"); html.append(value); html.append("</td>"); } html.append("</tr>"); } html.append("</table>"); FileOutputStream fos = new FileOutputStream("lib/output.html"); fos.write(html.toString().getBytes()); fos.close();提示错误Exception in thread "main" java.lang.NullPointerException at a.b.main(b.java:26)第26行是Cell cell = sheet.getRow(i).getCell(j);
这个错误通常是因为在获取行或单元格时,没有检查它们是否为 null 引用。你可以尝试在访问行或单元格之前先检查它们是否为 null,如果是 null,则可能是因为该行或单元格为空。以下是一个示例代码片段,可以用于检查并避免 NullPointerException 错误:
```
for (int i = 0; i < rowCount; i++) {
Row row = sheet.getRow(i);
if (row != null) {
html.append("<tr>");
for (int j = 0; j < colCount; j++) {
Cell cell = row.getCell(j);
if (cell != null) {
String value = cell.getStringCellValue();
html.append("<td style='width: " + cellWidth + "%; height: " + cellHeight + "%;'>");
html.append(value);
html.append("</td>");
}
}
html.append("</tr>");
}
}
```
在这个示例代码中,我们在访问每一行和单元格之前,都先检查了它们是否为 null 引用,如果是 null,则跳过该行或单元格。这样可以避免 NullPointerException 错误。
为这段代码解决Exception in thread "AWT-EventQueue-0" java.lang.ArrayIndexOutOfBoundsException: Index 4 out of bounds for length 4问题 public class readCproject { public Object[][] readProjectData(String ProjectParam) { Object[][] ProjectSum = new String[100][50]; for(int i=0;i<100;i++) { ProjectSum[i]=new String [50]; } try { // 文件 File file = new File(ProjectParam); // 判断如果文件不存在,则创建文件 if(!file.exists()) { try { file.createNewFile(); } catch (IOException e) { e.printStackTrace(); } } else{ BufferedReader br = new BufferedReader(new FileReader(file)); String s = null; int j = 0; while((s = br.readLine())!=null){ String[] strArray = s.split(","); System.out.println(strArray[0]); for(int l=0;l<4;l++) { ProjectSum[j][l]=strArray[l]; } j=j+1; } br.close(); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return ProjectSum; } }
在代码的第19行,你使用了一个数组索引变量l来访问strArray数组的元素。然而,在第20行中,你假设strArray数组的长度为4,但实际上并没有判断strArray数组的长度。因此,当strArray数组的长度小于4时,你将会遇到ArrayIndexOutOfBoundsException异常。
为了解决这个问题,你可以使用strArray.length来获取strArray数组的长度,并使用Math.min方法来确保不会超出数组的长度。修改第20行代码如下:
for(int l=0;l<Math.min(strArray.length, 4);l++)
{
ProjectSum[j][l]=strArray[l];
}
相关推荐
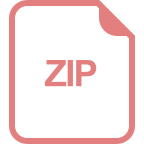
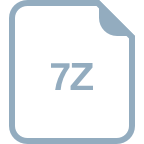
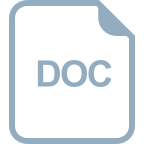













