图的邻接表表示法c++
时间: 2023-05-19 13:05:43 浏览: 131
邻接表是一种图的表示方法,它使用链表来存储每个节点的邻居节点。在C语言中,可以使用结构体和指针来实现邻接表。以下是一个示例代码:
```
#include <stdio.h>
#include <stdlib.h>
// 邻接表节点结构体
typedef struct AdjListNode {
int dest;
struct AdjListNode* next;
} AdjListNode;
// 邻接表头结构体
typedef struct AdjList {
AdjListNode* head;
} AdjList;
// 图结构体
typedef struct Graph {
int V;
AdjList* array;
} Graph;
// 创建邻接表节点
AdjListNode* newAdjListNode(int dest) {
AdjListNode* newNode = (AdjListNode*)malloc(sizeof(AdjListNode));
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
// 创建图
Graph* createGraph(int V) {
Graph* graph = (Graph*)malloc(sizeof(Graph));
graph->V = V;
graph->array = (AdjList*)malloc(V * sizeof(AdjList));
for (int i = 0; i < V; i++) {
graph->array[i].head = NULL;
}
return graph;
}
// 添加边
void addEdge(Graph* graph, int src, int dest) {
AdjListNode* newNode = newAdjListNode(dest);
newNode->next = graph->array[src].head;
graph->array[src].head = newNode;
newNode = newAdjListNode(src);
newNode->next = graph->array[dest].head;
graph->array[dest].head = newNode;
}
// 打印邻接表
void printGraph(Graph* graph) {
for (int i = 0; i < graph->V; i++) {
AdjListNode* node = graph->array[i].head;
printf("邻接表节点 %d 的邻居节点:", i);
while (node) {
printf("%d ", node->dest);
node = node->next;
}
printf("\n");
}
}
int main() {
Graph* graph = createGraph(5);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
printGraph(graph);
return 0;
}
```
这段代码实现了一个无向图的邻接表表示法,可以通过调用 `createGraph` 函数创建一个具有 `V` 个节点的图,然后通过 `addEdge` 函数添加边,最后通过 `printGraph` 函数打印邻接表。
相关推荐
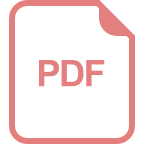
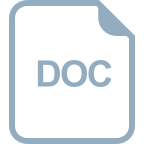














