使用C++语言,用动态规划算法求最长公共子序列,输出最长公共子序列的长度和最长公共子序列。
时间: 2024-10-31 15:07:10 浏览: 34
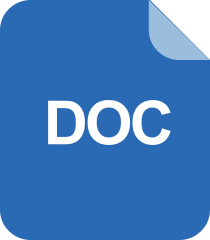
动态规划算法求最长公共子序列
在C++中,我们可以使用动态规划(Dynamic Programming)算法来解决最长公共子序列(Longest Common Subsequence, LCS)的问题。LCS是指两个序列中最长的共同部分,它不一定连续。
以下是使用二维数组实现的C++代码示例:
```cpp
#include <iostream>
#include <vector>
std::pair<int, std::string> longestCommonSubsequence(std::string str1, std::string str2) {
int m = str1.length();
int n = str2.length();
// 初始化二维数组 dp
std::vector<std::vector<int>> dp(m + 1, std::vector<int>(n + 1, 0));
// 动态规划填充过程
for (int i = 1; i <= m; ++i) {
for (int j = 1; j <= n; ++j) {
if (str1[i - 1] == str2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
dp[i][j] = std::max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
// 最终结果:最长公共子序列长度和子序列
int length = dp[m][n];
std::string lcs = "";
int i = m, j = n;
while (i > 0 && j > 0) {
if (str1[i - 1] == str2[j - 1]) {
lcs += str1[i - 1];
i--;
j--;
} else if (dp[i - 1][j] > dp[i][j - 1]) {
i--;
} else {
j--;
}
}
return {length, std::reverse(lcs.begin(), lcs.end())}; // 反转是因为我们从右上角开始填充,而我们需要左下角的结果
}
int main() {
std::string str1 = "ABCBDAB";
std::string str2 = "BDCAB";
auto result = longestCommonSubsequence(str1, str2);
std::cout << "Longest Common Subsequence Length: " << result.first << "\n";
std::cout << "Longest Common Subsequence: " << result.second << "\n";
return 0;
}
```
当你运行这个程序,会输出最长公共子序列的长度和子序列,例如对于输入 "ABCBDAB" 和 "BDCAB" ,结果可能是 "BCAB",长度为4。
阅读全文
相关推荐
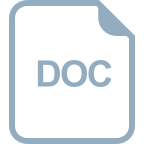
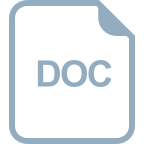















