python俄罗斯方块小游戏
时间: 2023-06-23 11:55:58 浏览: 49
好的,下面是一个简单的 Python 俄罗斯方块游戏实现:
```python
import random
import pygame
# 初始化 Pygame
pygame.init()
# 游戏窗口尺寸
WINDOW_WIDTH = 360
WINDOW_HEIGHT = 600
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义方块大小
BLOCK_SIZE = 30
# 定义方块类型和颜色
BLOCK_TYPES = [
((0, 0), (1, 0), (0, 1), (1, 1)), # 正方形
((0, 0), (1, 0), (2, 0), (3, 0)), # 棍子
((0, 0), (1, 0), (2, 0), (2, 1)), # L 形
((0, 0), (1, 0), (2, 0), (2, -1)), # 倒 L 形
((0, 0), (1, 0), (1, 1), (2, 1)), # Z 形
((0, 0), (1, 0), (1, -1), (2, -1)), # 倒 Z 形
((0, 0), (1, 0), (2, 0), (1, 1)), # T 形
]
BLOCK_COLORS = [
BLUE,
GREEN,
RED,
WHITE,
BLUE,
GREEN,
RED,
]
# 创建游戏窗口
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('俄罗斯方块')
# 创建字体
font = pygame.font.Font(None, 36)
# 定义游戏区域
game_area = [[0] * (WINDOW_WIDTH // BLOCK_SIZE) for _ in range(WINDOW_HEIGHT // BLOCK_SIZE)]
# 定义当前方块
current_block = None
# 定义当前方块位置
current_block_pos = None
# 定义计时器
clock = pygame.time.Clock()
current_time = 0
fall_time = 500
# 定义游戏结束标志
game_over = False
# 随机生成一个新方块
def new_block():
global current_block, current_block_pos
block_type = random.randint(0, len(BLOCK_TYPES) - 1)
current_block = BLOCK_TYPES[block_type]
current_block_pos = [WINDOW_WIDTH // BLOCK_SIZE // 2, 0]
# 检测方块是否超出边界或与已有方块重叠
def check_collision(block, pos):
for block_pos in block:
x, y = block_pos
x += pos[0]
y += pos[1]
if x < 0 or x >= len(game_area[0]) or y >= len(game_area) or game_area[y][x]:
return True
return False
# 将方块加入到游戏区域中
def add_block_to_game_area(block, pos):
for block_pos in block:
x, y = block_pos
x += pos[0]
y += pos[1]
game_area[y][x] = 1
# 消除满行
def remove_full_lines():
global game_area
new_game_area = []
num_lines_removed = 0
for row in game_area:
if 0 in row:
new_game_area.append(row)
else:
num_lines_removed += 1
new_game_area.insert(0, [0] * len(row))
game_area = new_game_area
return num_lines_removed
# 游戏主循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if not check_collision(current_block, [current_block_pos[0] - 1, current_block_pos[1]]):
current_block_pos[0] -= 1
elif event.key == pygame.K_RIGHT:
if not check_collision(current_block, [current_block_pos[0] + 1, current_block_pos[1]]):
current_block_pos[0] += 1
elif event.key == pygame.K_DOWN:
if not check_collision(current_block, [current_block_pos[0], current_block_pos[1] + 1]):
current_block_pos[1] += 1
elif event.key == pygame.K_UP:
# 旋转方块
rotated_block = []
for block_pos in current_block:
x, y = block_pos
x, y = y, -x
rotated_block.append((x, y))
if not check_collision(rotated_block, current_block_pos):
current_block = rotated_block
# 更新计时器
current_time += clock.tick()
# 方块下落
if current_time >= fall_time:
current_time = 0
if check_collision(current_block, [current_block_pos[0], current_block_pos[1] + 1]):
add_block_to_game_area(current_block, current_block_pos)
num_lines_removed = remove_full_lines()
if num_lines_removed:
print(f'{num_lines_removed} lines removed')
new_block()
if check_collision(current_block, current_block_pos):
game_over = True
else:
current_block_pos[1] += 1
# 绘制游戏区域
screen.fill(BLACK)
for y, row in enumerate(game_area):
for x, block in enumerate(row):
if block:
pygame.draw.rect(screen, BLOCK_COLORS[block % len(BLOCK_COLORS)], (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制当前方块
for block_pos in current_block:
x, y = block_pos
x += current_block_pos[0]
y += current_block_pos[1]
pygame.draw.rect(screen, BLOCK_COLORS[len(BLOCK_TYPES)], (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制分数
score = len(game_area) - game_area.count([0] * len(game_area[0]))
score_text = font.render(f'Score: {score}', True, WHITE)
screen.blit(score_text, (10, 10))
# 更新屏幕
pygame.display.update()
# 退出 Pygame
pygame.quit()
```
这个游戏是基于 Pygame 库实现的,需要先安装 Pygame 才能运行。
相关推荐
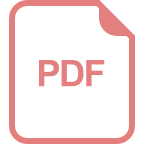










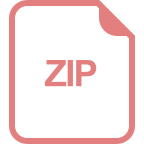
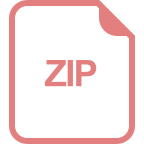
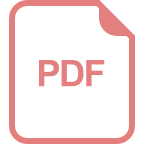
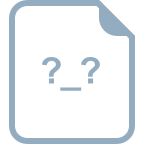